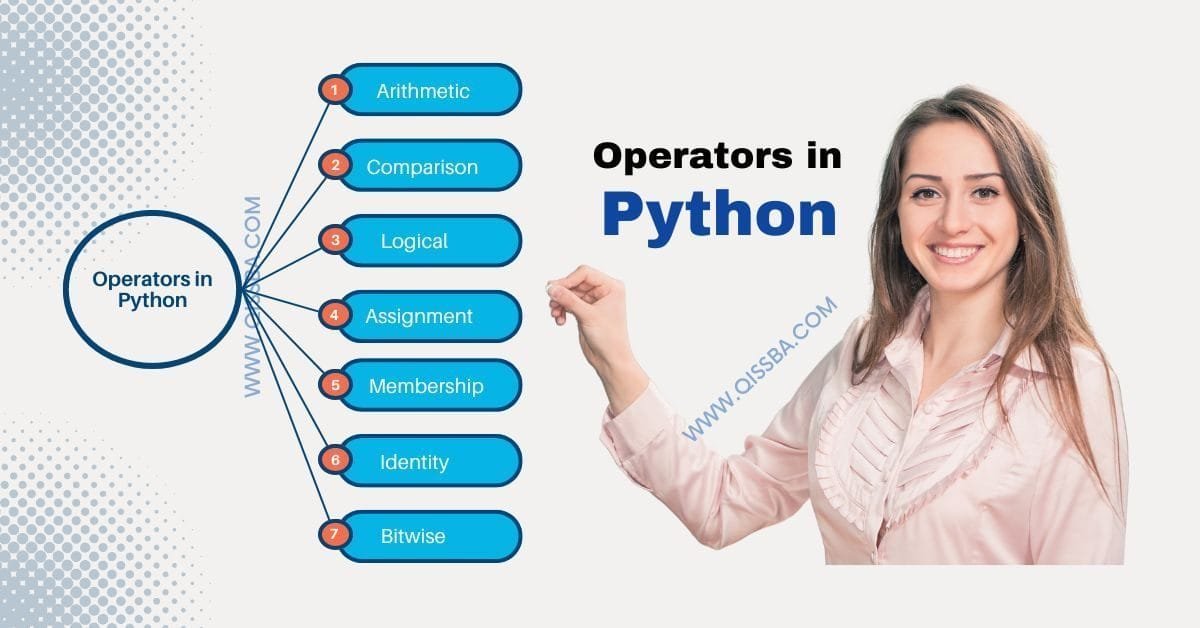
Operators in Python | CBSE Class 11| Computer Science
Dear Class 11th STUDENTS, ! Welcome to this tutorial of Operators in Python from your CBSE class 11 of Computer Science Syllabus.
In this tutorial, we shall be learning our chapter-4: Operators in Python from Unit 2: Programming and Computational Thinking (PCT-1) as CBSE BOARD suggested to learn about Operators in Python to complete this section.
After going through this tutorial, you will be able to understand the following topics from your latest syllabus 2025-26.
Unit 2: Programming and Computational Thinking (PCT-1)
Chapter 4: Operators in Python :
- Arithmetic operators
- Relational operators
- Logical operators
- Assignment operators
- Augmented assignment operators
- Identity operators (is, is not)
- Membership operators (in not in)
I advice you to check the latest syllabus given by CBSE Board at its Official website: www.cbseacademic.nic.in
Also, in this tutorial we will covers all necessary topics/concepts required to complete your exams preparations in CBSE classes 11th.
Also, you can Sign Up our free Computer Science Courses for classes 11th and 12th.
NOTE:
- We are also giving some important Questions & Answers for better understanding as well as preparation for your examinations.
- You may also download PDF file of this tutorial from our SHOP for free.
- For your ease and more understanding, we are also giving the video explanation class of each and every topic individually, so that you may clear your topics and get success in your examinations.
Introduction to Operators in Python
Operators in python are tokens that perform some computation (calculation / action) when they are applied to variable and other objects in an expression. Operators in python play an important role in data handling to furnish our objects for writing program logics and to get overall calculation results quickly.
In other way, we can say that operators are tokens that trigger some computation when applied to variables and other objects in an expression. Variables and other objects to which the computation is applied are called operands. It means an operator requires some operands to work upon.
The operations being carried out on data are represented by operators. The symbols that trigger the operation / action on data are called operators in python. The operations (specific tasks) are represented by operators and the objects of the operations are referred to as operands.
Types of Operators in Python:
Whenever, we required any mathematical computation like – addition, subtraction, etc, in our program logic, the python provides some operators to perform these operations in our python program.
However, in python. we can use many operators other than mathematics which are required, to perform computation to be applied for variable and other objects in an expression.
The following figure gives a brief description of the operators available in python.
Python has a rich set of operators comprises of these types of operators:
• Arithmetic operators
• Relational Operators
• Identity Operators
• Logical Operators
• Bitwise Operators
• Membership Operators.
• Comparison Operators
Let us discuss these python operators in detail one by one.
Arithmetic operators in Python:
To perform arithmetic operations, Python uses arithmetic operators. Python operators for basic calculations, as given below:
Each of these operators is a binary operator i.e., it requires two values (operands) to calculate a final answer. Apart from these binary operators, Python provides two unary arithmetic operators (that require one operand) also, which are unary +, and unary -.
Unary Operator
Unary (+)
The operators unary “+” precedes an operand. The operand (the value on which the operator operates) of the unary + operator must have arithmetic type and the result is the value of the argument. For example,
if a= 5 then + a means 5.
if a= 0 then + a means 0.
if a= -4 then + a means – 4.
Unary (-)
The operator unary – precedes an operand. The operand of the unary – operator must have arithmetic type and the result is the negation of its operand’s value. For example,
if a = 5 then – a means – 5.
if a = 0 then – a means 0 (there is no quantity known as – 0)
if a = -4 then – a means 4.
This operator reverses the sign of the operand’s value
Binary Operator
Operators that act upon two operands are referred to as Binary Operators. The operands of a binary operator are distinguished as the left or right operands. Together, the operator and its operands and its operands constitute an expression.
Addition Operator (+)
The arithmetic binary operator (Plus) + adds value of its operands and the result is the sum of the values of its two operands. For example,
4 +20 results in 24
a + 5(where a = 2) results in 7
a + b (where a =4, b = 6) result in 10
For Addition operator + operands may be of number types 5.
Python also offers + as a concatenation operator when used with strings, lists and tuples.
Subtraction Operator (-)
The (minus) – operator subtracts the second operand from the first. For example,
14- 3 evaluates to 11
a-b (where a =7, b=5) evaluates to 2
x-3 (where x = -1) evaluates to -4
The operands may be of number types.
Multiplication Operator (*)
The * operator multiplies the values of its operands. For example,
3*4 evaluates to 12
b*4(where b =6) evaluates to 24
p*2(where p = -5) evaluates to -10
a*c (where a =3, c=5) evaluates to 15
The operands may be of integer or floating-point number types.
Python also offers *as a replication operator when used with string.
Division Operator (/)
The / operator in Python 3.x divides its first operand by the second operand and always returns the result as a float value, e.g.,
4/2 evaluates to 2.0
100/ 10 evaluates to 10.0
7/2.5 evaluates to 2.8
100/32 evaluates to 3.125
13.5/1.5 evaluates to 9.0
Please note that in older version of Python (2.x) the / operator worked differently.
Floor Division Operator (//)
Python also offers another division operator //, which performs the floor division. The floor division is the division in which only the whole part of the result is given in the output and the fractional part is truncated.
To understand this, consider the third example of division given in division operator /, i.e,
a =15.9, b = 3,
a/b evaluates to 5.3.
Now if you change the division operator /, with floor division operator // in above expression, i.e,
Consider some more examples:
100/32 evaluates to 3.0
7/ /3 evaluates to 2
6.5/2 evaluates to 3.0
The operands may be of number types.
Modulus Operator (%)
The % operator finds the modulus (i.e, Remainder but pronounced as mo-du-lo) Of its first operand relative to the second. That is, it produces the remainder of dividing. The first operand by the second operand.
For example,
19% 6 evaluates to1, since 6 goes into 19
Three times with a remainder1.
Similarly,
7.2%3 will yield 1.2
6 %2.5 will yield 1.0
The operands may be number types.
Exponentiations Operator (**)
The exponentiation operator ** performs exponentiation (power) calculation, i.e., it returns the result of a number raised to a power exponent.
For example,
4**3 evaluates to 64(43)
a**b (a=7, b = 4) Evaluates to 2401 (ab i.e., 74).
x**0.5 (x = 49.0) evaluates to 7.0 (x0.5, i.e, x, i.e, 49)
27.009 ** 0.3 evaluates to 2. 68814413570761(27. 0090.3
The operands may be number types.
Example of Exponentiations Operator
Prints the area of a circle of radius 3.75 meters.
Solution.
Radius = 3.75
Area = 3.14159 * * 2
Print (Area, ’Sq. meter’)
Negative Number Arithmetic in Python
Arithmetic operations are straightforward even with negative numbers, especially with non-division operators i.e.
-5 + 3 will give you 2
-5 – 3 will give you -8
-5 * 3 will give you -15
-5 ** 3 will give you -125
But when it comes to division and related operators (/, //, %), mostly people get confused. Let us see how python evaluates theses. To understand this, we recommend that you look at the operation shown in the adjacent screenshot and then look for its working explained below, where the result is shown shaded.
Relational Operators in Python:
In the term relational operator, relational refers to the relationships that values (or operands) can have with one another. Thus, the relational operators determine the relation among different operands. Python provides six relational operators for comparing values (thus also called comparison). If the comparison is true, the relational expression results into the Boolean value True and to Boolean value False, if the comparison is false.
The six relational operators are:
Relational operators work with nearly all types of data in Python, such as numbers, strings, lists, tuples etc.
Relational operators work on following principles:
- For numeric types, the values are compared after removing trailing zeros after decimal point from a floating-point number. For example,4 and 4.0 will be treated as equal (after removing trailing zeros from 4.0, it becomes equal to 4 only).
- Strings are compared on the basis of lexicographical ordering (ordering in dictionary).
- Capital letters are considered lesser than small letters, e.g., ‘A’ is less than ‘a’; ‘Python’ is not equal to ‘python’; ‘book’ is not equal to ‘books.
Lexicographical ordering is implemented via the corresponding codes or ordinal values (ASCII code or Unicode code) of the characters being compared. That is the reason ‘A’ is less than ‘a’ because ASCII value of letter ‘A’ (65) is less than ‘a’ (97). You can check for ordinal code of a character yourself using Ord(<character>) function (for example Ord(‘A’) ) .
- For the same reason, you need to be careful about nonprinting characters like spaces. Spaces are real characters and they have a specific code (ASCII code 32) assigned to them. If you are comparing two strings that appear same to you but they might produce a different result – if they have some spaces in the beginning or end of the string. See example below.
- Two lists and similarly two tuples are equal if they have same elements in the same order.
- Boolean True is equivalent to 1 (numeric one) and Boolean False to 0 (numeric zero) comparison purposes.
For instance, consider the following relational operations as given below.
a<b will return True
c < d will return False
f < h will return False
f = = h will return True
C == e will return False
g ==j will return True
‘’God’’ < ‘’Godhouse’’ will return True
# Both match up to the letter ‘d’ but ‘God’ is shorter than ‘Godhouse’ so it comes first in the dictionary.
‘’god’’ < ‘’Godhouse’’ will return False
# The length of the strings does not matter here. Lower case g is greater than upper case G.
a ==p will return True
L== M will return False
L == N will return True
O == P will return False
O == Q will return True
a = = True will return False
O == False will return True
1 = = True will return True
While using floating-point numbers with relational operators, you should keep in mind that floating point numbers are approximately presented in memory in binary from up to the unexpected results if you are comparing floating-point numbers especially for equality (=). Number such as 1/3 etc., cannot be fully represented as binary as it yields 0.3333… etc. and to represent it in binary some approximation is done internally.
Consider the following code for to understand it:
Thus, you should avoid floating point equality comparisons as much as you can.
Identity Operators in Python:
There are two identity operators in python is and is not. The identity operators are used to check if both the operands reference the same object memory i.e., the identity operators compare the memory location of two objects and return true or false accordingly.
Consider the following examples:
A = 10
B = 10
A is B
will return True because both are referencing the memory address of value 10
you can use id () to confirm that both are referencing same memory address.
Now if you change the value of b so that it is not referring to same integer object, then expression a is b will return True
The is not operator is opposite of the is operator. It returns True when both its operands are not referring to same memory address.
Equality (= =) and identity (is) – Important Relation
You have seen in above given examples that when two variables are referring to same value, the is operator returns True. When the is operator returns True for two variables, it implicitly means that the equality operator will also return True. That is, expression a is b as a True means that a = b will also be True, always. See below:
In [58]: print (a, b)
235 235
In [59]: a is b
Out [59]: True
In [60]: a == b
Out [60]: True
But it is not always true other way round. That means there are some cases where you will find that the two objects are having just the same value i.e., = operator returns True for them but the is operator returns False.
See in the screenshots shown here.
Similarly
Also
The reason behind this behavior is that there are a few cases where python creates two different objects that both store the same value. These are:
- Input of strings from the console;
- Writing integers literals with many digits (very big integers);
- Writing floating – point and complex literals.
Following figure illustrates one of the above given screenshots.
Most of the times we just need to check whether the two objects refer to the same value or not – in this case the equality operator (==) is sufficient for this test. However, in advanced programs or in your projects, you may need to check whether they refer to same memory address or not – in this case, you can use the is operator.
Augmented Assignment Operators
You have learnt that python has an assignment operator = which assigns the value specified on RHS to the variable/ object on the LHS of = python also offers augmented assignment arithmetic operators, which combine the impact of an arithmetic operator with an assignment operator, e.g., if you want to add value of b to value of a and assign the result to a, then instead of writing
a = a + b
You may write
a + = b
To add value of a to value of b add assign the result to b, you may write
b + = a # instead of b= b+ a
These operators can be used anywhere that ordinary assignment is used. Augmented assignment doesn’t violate mutability. Therefore, writing x + = y creates an entirely new object x with the value x + y.
Membership Operator in Python:
A membership operator in Python is used to test whether a value or element is present in a sequence (like a list, tuple, string, set, or dictionary) or not. There are two membership operators: in and not in.
Membership Operators:
- in: Returns True if the value is found in the sequence, otherwise False.
- not in: Returns True if the value is not found in the sequence, otherwise False.
Syntax:
- value in sequence # Checks if value is present in sequence
value not in sequence # Checks if value is not present in sequence
Examples with Lists:
my_list = [1, 2, 3, 4, 5]
print(3 in my_list) # Output: True
print(6 in my_list) # Output: False
print(6 not in my_list) # Output: True
Examples with Strings:
my_string = “Hello, World!”
print(“Hello” in my_string) # Output: True
print(“Python” in my_string) # Output: False
print(“Python” not in my_string) # Output: True
Examples with Tuples:
my_tuple = (10, 20, 30, 40)
print(20 in my_tuple) # Output: True
print(50 not in my_tuple) # Output: True
Examples with Sets:
my_set = {1, 2, 3}
print(2 in my_set) # Output: True
print(4 not in my_set) # Output: True
Examples with Dictionaries:
For dictionaries, in checks for the presence of keys (not values).
my_dict = {“a”: 1, “b”: 2, “c”: 3}
print(“a” in my_dict) # Output: True
print(“d” in my_dict) # Output: False
print(“d” not in my_dict) # Output: True
Logical operators in Python:
An earlier section discussed about relational operators that establish relationships among the values. This section talks about logical operators, the Boolean logical operators (or, and, not) that refer to the ways these relationships (among values) can be connected. Python provides three logical operators to combine existing expressions. There are or, and, and not.
Before we proceed to the discussion of logical operators, it is important for you to know about Truth value testing, because in some cases logical operators base their results on truth value testing.
Truth value Testing
Python associates with every value type, some truth value (the truthiness), i.e. python internally categorizes them as true or false. Any object can be tasted for truth value. Python considers following values false, (i.e., with truth – value as false) and true:
Do not confuse between Boolean values True, false and truth values (truthiness values) true, false. simply put truth – value tests for zero – ness or emptiness of a value. Boolean values belong to just one data type, i.e., Boolean type, where as we can test truthiness for every value object in python. But to avoid any confusion, we shall be giving truth values true and false in small letters and with a subscript tval, i.e., now on this chapter true tval and false tval will be referring to truth- values of an object.
The utility of Truth testing will be clear to you as we discussing the functioning of logical operators.
The or Operator
The or operator combines two expressions, which make its operands. The or operator works in these ways:
- Relational expressions as operands
- Numbers or string or lists as operands
Relational expressions as operands
When or operator has its operands as relational expression (e. g., p > q, j! = k, etc.) then the or operator performs as per following principal:
The or operator evaluates to True if either of its (relation) operands evaluates to True; False if both operands evaluate to false.
That is:
Following are some examples of this or operation:
Numbers / string / lists as operands
When or operator has its operands as numbers or string or lists (e.g., ‘a’ or’’, 3 or o, etc.) then the or operator performs as per following principal:
In an expression x or y, if first operand, (i. e., expression x) has false tval, then return second operand y as result, otherwise return x.
That is:
Examples
How the truth value is determined? – refer to section above.
The or operator will test second operand only if the first operand is false, otherwise ignore it; even if the second operand is logically wrong e.g.,
20 > 10 or “a” +1>1
Will give you result as
True
Without checking the second operand of or i.e., “a” + 1 > 1, which is syntactically wrong – you cannot add an integer to a string.
The and operator
The and operator combines two expressions, which make its operands. The and operator works in these ways:
- Relational expressions as operands
- Numbers or string or lists as operands
Relational expressions as operands
When and operator has its operands as relational expression (e. g., p > q, j! = k, etc.) then the and operator performs as per following principal:
The and operator evaluates to True if both of its (relational) operands evaluates to True; False if either or both operands evaluate to false.
That is
Following are some examples of this and operation:
Numbers / string / lists as operands
When and operator has its operands as numbers or string or lists (e.g., ‘a’ or’’, 3 or o, etc.) then the and operator performs as per following principal:
In an expression x and y, if first operand, (i.e., expression x) has false tval, then return first operand x as result, otherwise return y.
That is.
Example
How the truth value is determined? – refer to section above.
Please Note:-
The and operator will test second operand only if the first operand is false, otherwise ignore it; even if the second operand is logically wrong e.g.,
10> 20 and “a” +10 < 5
Will give you result as
False
Ignoring the second operand completely even if it is wrong –you cannot add an integer to a string in python.
The not operator
The Boolean / logical not operator, works on single expression or operand i.e., it is a unary operator. the logical not operator negates or reverse the truth value of the expression following it i.e., if the
expression is True or true tval, then not expression is false, and vice versa.
unlike ‘and’ and ‘or’ operators that can return number or a string or a list etc. as result, the ‘not’ operator returns always a Boolean value True or False.
Consider same examples:
Following table summarizes the logical operators.
Chained comparison operators
While discussing logical operators, python has something interesting to offer. You can chain multiple comparisons which are like shortened version of larger Boolean expressions. Let us see how. Rather than writing 1 < 2 and 2< 3, you can even write 1 < 2 < 3 which the chained version of earlier Boolean expression.
The above statement will check if 1 was less than 2 and if 2 was less than 3
Let’s look a a few examples of using chains:
>>> 1 < 2 < 3 is equivalent to >>> 1< 2 and 2 < 3
True True
As per the property of and, the expression 1< 3 will be first evaluated and if only it is True, then only the next chained expression 2< 3 will be evaluated.
Similarly consider some more examples:
>>> 11 < <13 > 12
True
The above expression checks if 13 is larger than both the numbers: it is the shortened version of 11 < 13 and 13 > 12.
Bitwise Operators in Python:
Python also provides another category of operators- bitwise operators, which are similar to the logical operators, except that they work on a smaller scale- on binary representations of data.
Bitwise operators are used to change individual bits in an operand.
Python provides following Bitwise operators.
Table of Bitwise operators
The Bitwise AND operator (&)
When its operands are numbers, the & operation performs the bitwise AND function on each parallel pair of bits in each operand. The AND function sets the resulting bit 1 if the corresponding bit in both operands is 1, as shown in the following table.
Suppose that you were to AND the values 13 and 12, like this: 13 & 12. The result of this operation is 12 because the binary representation of 13 is 1100, and the binary representation of 13 is 1101. You can use bin () to get binary representation of a number.
If both operand bits are 1, the AND functions set the resulting bit to 1; otherwise, the resulting bit is 0. So, when you line up the two operands and perform the AND function, you can see that the two high-order bits (the two bits farthest to the left of each number) of each operand are 1. Thus, the resulting bit in the result is also 1. The low- order bits evaluate to 0 because either one or both bits in the operands are 0.
The inclusive OR operator (|)
When both of its operands are numbers, the | operator performs the inclusive OR operation. Inclusive OR means that if either of the two bits is 1, the result is 1. The following Table.
Shows the results of inclusive OR operations.
For OR operations, o OR O produces o. Any other combination produces 1.
The exclusive OR (XOR) operator (^)
Exclusive OR means that if the two operand bits are different, the result is 1; otherwise, the result is 0. The following table shows the results of an exclusive OR operation.
For XOR operations, 1 XOR produces 1, as does 0 XOR 1. (All these operations are commutative.) Any other combination produces 0.
The Complement Operator (~)
The complement operator inverts the value of each bit of the operand: if the operand bit is 1 the result is 0 and if the operand bit is 0 the result is 1.
——————————————————————————————————————————————————–
Exam Time
Chapter : Python Operators
IMPORTANT QUESTIONS : Asked in examinations of Class 12th Computer Science
IMPORTANT QUESTIONS
Q-1. What are python operators ?.
Q-2. What are types of python operators ?.
Q-3. What are the functions of operators?
Q-4. What are 7 types of python operators ?.
Q-6. How many types of operators used in python ?
Read more topics related to Operators in Python
Operator Precedence CLICK HERE
Operator Associativity CLICK HERE