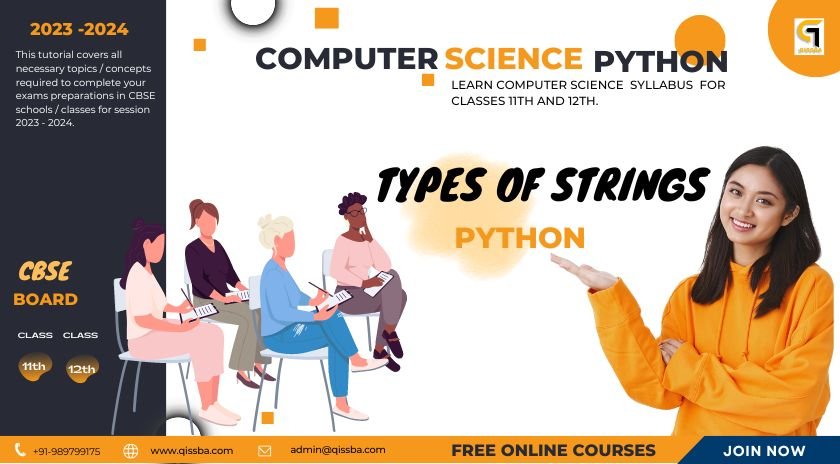
Types of Strings in Python | CBSE – Class 12
In this section “Exploring the Different Types of Strings in Python: types and their functions“, we will provide a comprehensive introduction to Different Types of Strings in Python with examples including
- Single Line Strings.
- Multiline Strings.
- ASCII Strings.
- Unicode Strings.
- Raw Strings.
- Formatted Strings.
- Byte Strings.
And, by the end of this tutorial, readers will have a solid understanding of all string types in Python and will be able to use this knowledge in their own programming projects.
Also this tutorial covers all necessary topics/concepts required to complete your exams preparations in CBSE schools / classes 11th and 12th.
Introduction:
Python is a powerful programming language that is widely used for developing web applications, scientific computing, data analysis, and artificial intelligence projects.
Here, in python, One of the fundamental data types in Python is the string, which is a sequence of characters enclosed in single or double quotes.
And, we know that strings are one of the most commonly used data types in Python. They are used to store text data and are represented as a sequence of characters.
Let’s start exploring the different types of strings in Python and their functions.
Type 1: Single Line Strings:
Single line strings are strings that are contained within a single line. They are the most commonly used type of string in Python and are created by enclosing a sequence of characters within single or double quotes.
Here’s an example of creating a single line string:
my_string = "Hello, World!"
In this case, my_string is a single line string that contains the text “Hello, World!”.
Single line strings are useful for storing short text strings such as variable names, function names, and short messages. They are also used for concatenating with other strings and for performing string operations.
Multiline Strings:
Multiline strings are strings that span across multiple lines. They are created by enclosing a sequence of characters within triple quotes – either single or double quotes. Multiline strings are useful when you need to store long text strings that span across multiple lines, such as paragraphs, articles, or code comments.
Here’s an example of creating a multiline string:
my_string = '''Python is a powerful programming language that is used for web development, data analysis, machine learning, and artificial intelligence. It has a simple and easy-to-learn syntax and is loved by developers worldwide.'''
In this case, my_string is a multiline string that contains the text “Python is a powerful programming language that is used for web development, data analysis, machine learning, and artificial intelligence. It has a simple and easy-to-learn syntax and is loved by developers worldwide.”.
Multiline strings are useful for creating complex string structures such as formatted strings, code blocks, and documentation strings. They are also useful for storing text that needs to be formatted or indented.
ASCII Strings:
The ASCII string is the most basic type of string in Python. It contains only the characters in the ASCII character set, which includes letters, numbers, and symbols commonly used in the English language. You can create an ASCII string in Python by enclosing the characters in single or double quotes.
Here’s an example:
my_string = 'Hello, World!'
In this case, my_string is an ASCII string that contains the text “Hello, World!”.
Unicode Strings:
Unicode is a standard for encoding characters from all the world’s writing systems, including languages that use non-Latin scripts. Python supports Unicode strings, which can represent characters from any language or writing system.
You can create a Unicode string in Python by adding the prefix “u” or “U” before the quotes:
my_unicode_string = u'Hello, 世界!'
In this case, my_unicode_string is a Unicode string that contains the text “Hello, 世界!”, which means “Hello, world!” in Chinese.
Raw Strings:
A raw string is a string that is treated as a sequence of characters without any special meaning for backslashes. This is useful for strings that contain regular expressions, file paths, or other characters that may be interpreted differently in Python.
You can create a raw string in Python by adding the prefix “r” or “R” before the quotes:
my_raw_string = r'C:\Users\Documents\file.txt'
In this case, my_raw_string is a raw string that represents the file path C:\Users\Documents\file.txt”.
Formatted Strings:
Formatted strings are a convenient way to insert variables and expressions into a string. You can create a formatted string in Python by adding the prefix “f” or “F” before the quotes and using curly braces to enclose the variables or expressions:
name = 'Alice' age = 30 my_formatted_string = f'My name is {name} and I am {age} years old.'
In this case, my_formatted_string is a formatted string that contains the variables name and age in the text.
Byte Strings:
Byte strings are a type of string that contains bytes instead of characters. This is useful for working with binary data, such as images, audio, or video files. You can create a byte string in Python by adding the prefix “b” or “B” before the quotes:
my_byte_string = b'\x48\x65\x6c\x6c\x6f\x20\x57\x6f\x72\x6c\x64\x21'
In this case, my_byte_string is a byte string that contains the hexadecimal representation of the ASCII string “Hello World!”.
Conclusion:
In this post, firstly, we’ve explored the two basic types of strings in Python as– single line and multiline strings – and their functions.
Single line strings are useful for storing short text strings, while multiline strings are useful for storing long text strings that span across multiple lines.
Secondly, we’ve explored the some other advance types of strings in Python as ASCII strings, Unicode strings, raw strings, formatted strings, and byte strings.
By understanding the differences between above discussed strings, you can choose the best string type for your Python projects and perform string operations.