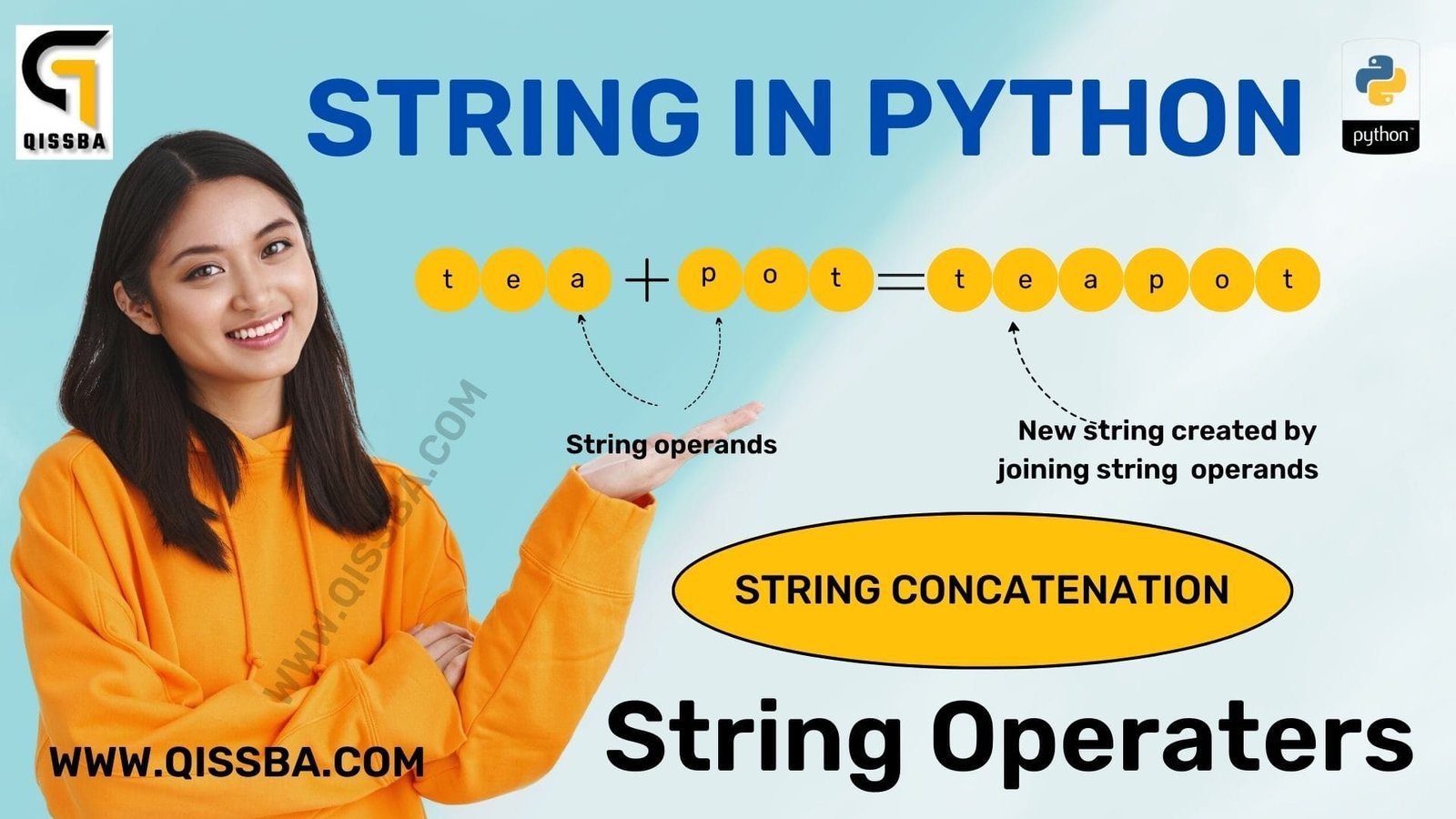
String Concatenation in Python | CBSE – Class 12
In this section, “Strings Concatenation in Python: How to Join Strings Together”, we will provide a comprehensive introduction to Concatenation of strings in python, including
- Basic String Concatenation method.
- By using join() Method
- By using formatted string literals.
- Best Practices for String Concatenation
By the end of this tutorial, readers will have a solid understanding of string Concatenation in Python and will be able to use this knowledge in their own programming projects.
And this tutorial covers all necessary topics/concepts required to complete your exams preparations in CBSE schools / classes.
Introduction:
In Python, strings are a fundamental data type used to represent text. String concatenation is the process of joining two or more strings together to create a single string. This operation is essential when working with text data in Python, and it’s a topic that every Python developer should be familiar with. In this article, we’ll explore how to concatenate strings in Python, the different methods available, and best practices to ensure optimized performance.
Basic String Concatenation in python:
The most common way to concatenate strings in Python is by using the + operator. For example: like
string1 = "Hello"
string2 = "World"
result = string1 + string2
print(result)
Output: HelloWorld
So, In this example, we’ve created two strings, “Hello” and “World,” and joined them together using the + operator. The resulting string is “HelloWorld.”
String Concatenation using join() Method
Another way to concatenate strings in Python is by using the join() method. The join() method is a string method that takes an iterable as an argument and returns a string. For example: like
words = ["Hello", "World"]
result = " ".join(words)
print(result)
Output: Hello World
In this example, we’ve created a list of two strings, “Hello” and “World,” and joined them together using the join() method. The resulting string is “Hello World.”
Formatting Concatenation in python:
Python also provides a third way to concatenate strings, which is by using formatted string literals (f-strings). F-strings are a convenient and concise way to embed variables and expressions inside a string. For example: like
name = "John"
age = 25
result = f"My name is {name} and I am {age} years old."
print(result)
Output: My name is John and I am 25 years old.
Now, In this example, we’ve used an f-string to create a sentence that includes variables “name” and “age.” The resulting string is “My name is John and I am 25 years old.”
Best Practices for String Concatenation in python:
When working with strings in Python, it’s essential to follow some best practices to ensure optimized performance. Here are some tips to keep in mind:
- Use the join() method instead of the + operator when joining many strings.
- And avoid using the + operator to concatenate strings in a loop. This operation is inefficient and can cause performance issues. Instead, use the join() method or create a list of strings and join them at once.
- And use f-strings for formatted string concatenation. They are more readable and efficient than other methods.
Conclusion:
In this article, we’ve explored the different ways to concatenate strings in Python, including the + operator, join() method, and f-strings. We’ve also provided some best practices to follow to ensure optimized performance. By understanding the basics of string concatenation, you’ll be able to work with text data more efficiently and write better Python code.