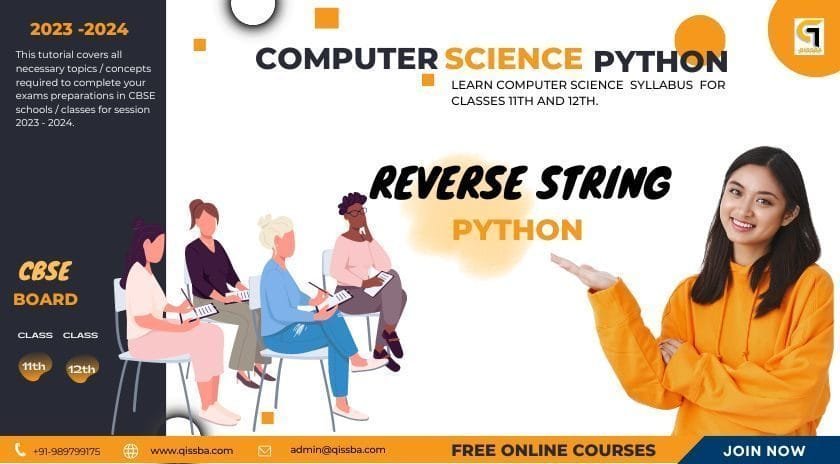
Reverse String in Python | CBSE – Class 12
In this section, “Reverse String in Python: How to Flip Your Text”, we will provide a comprehensive introduction to replacing strings in python, including
- Use slicing to reverse a string
- Using the reversed() function
- Using a loop to reverse a string
- Best Practices for String Reversal
By the end of this tutorial, readers will have a solid understanding of Reversing strings in Python and will be able to use this knowledge in their own programming projects.
This tutorial covers all necessary topics/concepts required to complete your exams preparations in CBSE schools / classes.
Introduction:
Reversing a string is a common operation when working with text data in Python. In this article, we’ll explore how to reverse a string in Python and the various methods available for flipping your text.
Using slicing to reverse a string:
One straightforward way to reverse a string in Python is by using slicing. Slicing is a way to extract a portion of a string by specifying start and end indices. Here’s an example of how to use slicing to reverse a string:
string = "Hello World"
new_string = string[::-1]
print(new_string)
Output: ‘dlroW olleH’
With this example, we’ve used slicing to extract the entire string, but with a step of -1. This means that we’re starting at the end of the string and moving backward by one character at a time.
Using the reversed() function:
Another way to reverse a string in Python is by using the built-in reversed() function. This function takes an iterable object, such as a string or a list, and returns a reverse iterator. Here’s an example:
string = "Hello World"
new_string = ''.join(reversed(string))
print(new_string)
Output: ‘dlroW olleH’
In this example, we’ve used the reversed() function to create a reverse iterator for the string. We then used the join() method to join the characters back together into a new string.
Using a loop to reverse a string:
You can also reverse a string in Python by using a loop to iterate through the characters and build a new string in reverse order. Here’s an example:
string = "Hello World"
new_string = ""
for char in string:
new_string = char + new_string
print(new_string)
Output: ‘dlroW olleH’
With this example, we’ve used a loop to iterate through the characters of the string and build a new string in reverse order by concatenating each character to the beginning of the new string.
Best Practices for String Reversal:
When working with string reversal in Python, it’s essential to follow some best practices to ensure optimized performance. Here are some tips to keep in mind:
- Use slicing to reverse a string for the most efficient and concise solution.
- Use the reversed() function when working with other iterable objects, such as lists or tuples.
- Be careful when reversing strings in loops. This can be computationally expensive and slow down your code.
Conclusion:
In this article, we’ve explored how to reverse a string in Python using slicing, the reversed() function, and a loop. By following best practices for string reversal, you’ll be able to manipulate string data more efficiently and write better Python code.