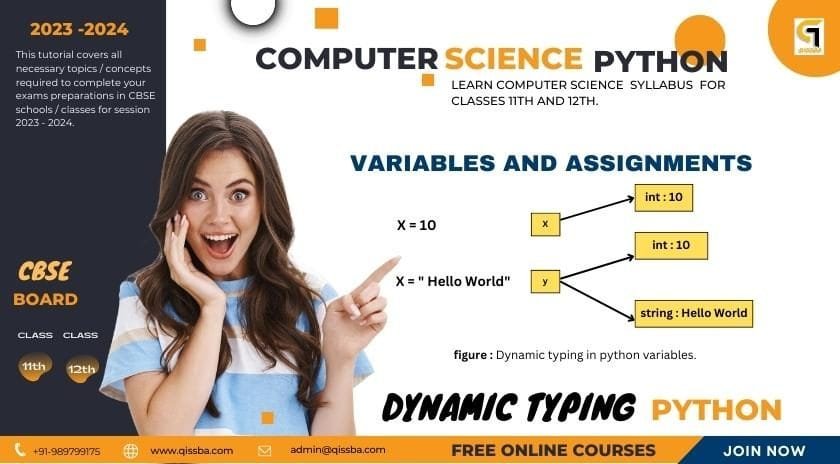
Dynamic Typing in Python CBSE – Class 12
In this section “Dynamic Typing in Python“, we will provide a comprehensive introduction to working with Dynamic Typing in Python with examples including
- What is Dynamic Typing in Python.
- Example of Dynamic Typing in Python.
- Static typing VS Dynamic typing.
- Advantages of Dynamic Typing in Python.
- Disadvantages of Dynamic Typing in Python.
- Why Python is called Dynamically Typed?
And, by the end of this tutorial, readers will have a solid understanding of all about Dynamic Typing in Python and will be able to use this knowledge in their own programming projects.
Also this tutorial covers all necessary topics/concepts required to complete your exams preparations in CBSE schools / classes 11th and 12th.
Also , you can Sign Up our free Computer Science Courses for classes 11th and 12th.
What is Dynamic Typing in Python?
Dynamic typing is a type system used in programming languages, including Python, where variables are not explicitly assigned a data type. Instead, the data type is inferred at runtime based on the value assigned to the variable.
Python is a dynamically typed programming language, which means that the types of variables are determined at runtime, rather than being declared explicitly in code. In this article, we will explore the concept of dynamic typing in Python, its advantages and disadvantages, and how it differs from static typing.
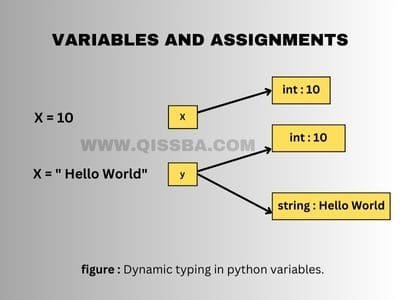
Dynamic Typing Example
In python, as you have learnt, a variable is defined by assigning to it some value (of a particular type such as numeric, string etc.)
X=10
We can say that variable x is referring to a value of integer type.
Later in your program, if you reassign a value of some other type to variable x, python will not complain (no error will be raised), e.g.
X=10
Print(x)
X=’’Hello World”
Print(x)
Above code will yield the output as:
10
Hello World
So, you can think of python variables as labels associated with objects (literal values in our case here); with dynamic typing, python makes a label refer to new value with new assignment the following figure illustrates it.
In both cases, the data type of x is determined at runtime based on the value assigned to it. This allows for more flexibility in programming, as variables can be assigned any value without needing to declare a specific data type.
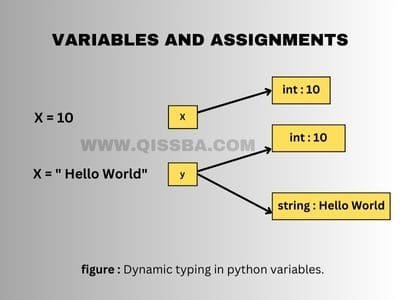
As you can see in figure variable X is first pointing to/referring to an integer value 10 and then to a string value “HELLO WORLD “.
Please not hear that variable X does not have a type but the value it points to does have a type. so, you can make a variable point to a value of different type by reassigning a value of that type; python will not raise any error. This is called dynamic typing feature of python.
However, it also means that programmers need to be careful when assigning values to variables, as it is possible to accidentally assign an incorrect data type, which can cause errors later in the code. Therefore, it’s important to use good coding practices and testing to ensure that your code is working as intended.
Static Typing v/s Dynamic Typing
The main difference between static and dynamic typing in Python is in how they handle variable types.
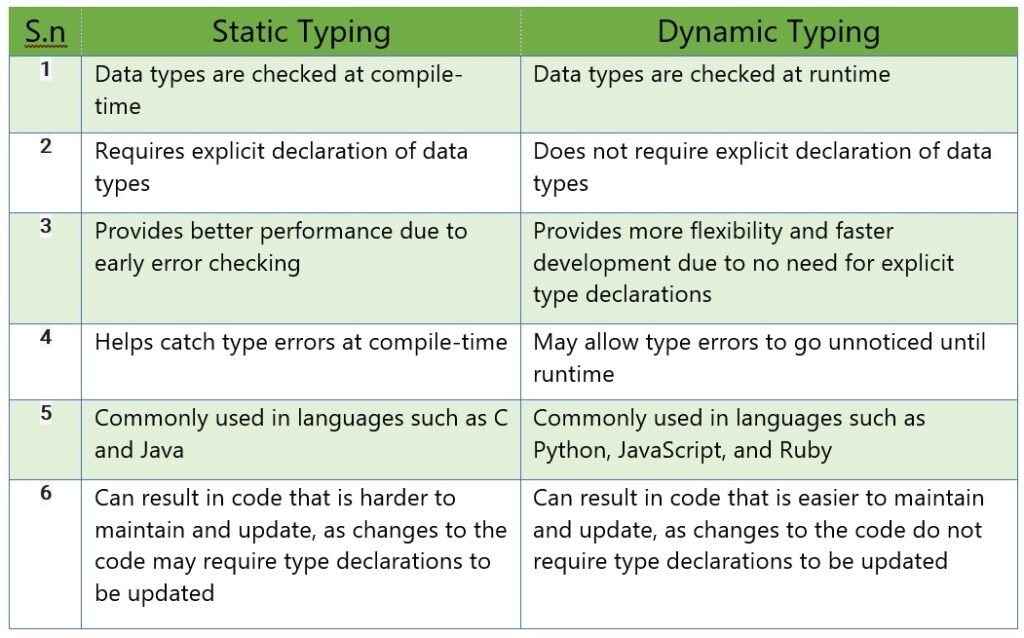
Static typing: is a type system where variables must be explicitly declared with a specific data type before they can be used. The data type of a variable cannot be changed once it is declared. The purpose of static typing is to catch errors early on in the development process, before the program is executed.
Dynamic typing: on the other hand, is a type system where variables do not need to be explicitly declared with a specific data type. The data type of a variable is determined at runtime based on the value assigned to it. The purpose of dynamic typing is to provide more flexibility in programming and to make it easier to write and maintain code.
Here are some key differences between static and dynamic typing in Python:
Declaration: In static typing, variables must be declared with a specific data type before they can be used. In dynamic typing, variables do not need to be declared with a specific data type.
Type Checking: In static typing, the type of a variable is checked at compile-time. In dynamic typing, the type of a variable is checked at runtime.
Flexibility: Static typing provides less flexibility in programming, as variables must be explicitly declared with a specific data type. Dynamic typing provides more flexibility in programming, as variables can be assigned any value without needing to declare a specific data type.
Ease of Use: Dynamic typing can be easier to use, as it reduces the amount of boilerplate code needed. However, it can also make it harder to catch errors, as variable types are not explicitly defined.
Performance: Static typing can be faster than dynamic typing, as the type of a variable is determined at compile-time, rather than runtime. However, the difference in performance may not be noticeable in small or simple programs.
Conclusion: In Python, dynamic typing is the default type system. However, Python also allows for static typing through the use of type annotations, which allow you to declare the data type of a variable or function parameter. This feature was introduced in Python 3.5 and can be used to catch errors early on in the development process.
Static typing is used in languages such as C and Java, where data types are checked at compile-time and require explicit declaration.
This provides better performance due to early error checking and helps catch type errors at compile-time.
However, it can result in code that is harder to maintain and update, as changes to the code may require type declarations to be updated.
Dynamic typing is used in languages such as Python, JavaScript, and Ruby, where data types are checked at runtime and do not require explicit declaration.
This provides more flexibility and faster development due to no need for explicit type declarations, but may allow type errors to go unnoticed until runtime.
It can also result in code that is easier to maintain and update, as changes to the code do not require type declarations to be updated.
Overall, the choice between static typing and dynamic typing depends on the requirements of the application, the language being used, and the tradeoff between performance and flexibility.
Advantages of Dynamic Typing in Python
Benefits or Advantages:
One of the main advantages of dynamic typing is that it allows for more flexibility in programming.
Since variables don’t need to be declared with a specific data type, programmers can easily switch between data types, without needing to modify the code.
This makes it easier to write and maintain code, as it reduces the amount of boilerplate code needed.
Two: Dynamic typing also enables type inference, which means that the interpreter automatically determines the data type of a variable based on the value assigned to it.
This can save time and reduce errors, as programmers don’t need to explicitly define the data type for each variable.
Three: Another advantage of dynamic typing is the ability to use duck typing, which means that the type of an object is determined by its behavior, rather than its class or type.
This can be useful when working with objects that have similar behaviors, but different classes or types.
Disadvantages of Dynamic Typing in Python
Drawbacks or Disadvantages:
One of the main disadvantages of dynamic typing is that it can make it harder to catch errors. Since variable types are not explicitly defined, it is possible to assign an incorrect data type to a variable, which can cause errors later in the code.
This can be mitigated by using good coding practices and testing, but it is still a risk.
Two: Dynamic typing can also be slower than static typing, as the interpreter needs to determine the data type of each variable at runtime.
This can result in longer execution times for large or complex programs.
Dynamically Typed language: Python
Why Python is called Dynamically Typed?
Python is called dynamically typed because it is a programming language that supports dynamic typing. Dynamic typing is a type system where variables do not need to be explicitly declared with a specific data type, and the data type of a variable is determined at runtime based on the value assigned to it.
Exam Time
Here are some important questions and answers on the topic of Dynamic Typing in Python:
Q. How can developers ensure code quality with dynamic typing in Python?
While dynamic typing can make code more flexible and adaptable, it’s important for developers to take steps to ensure code quality and minimize potential errors. Some best practices for working with dynamic typing in Python include:
- Writing clear and concise code: Clear and concise code can make it easier to understand the data types of variables and reduce the potential for errors.
- Using descriptive variable names: Descriptive variable names can help developers keep track of the data types of variables and avoid confusion.
- Adding comments and documentation: Comments and documentation can provide additional context and clarity about the data types of variables and how they are being used in the code.
- Writing unit tests: Unit tests can help catch errors related to data types and ensure that code functions correctly under different scenarios and data types.
Q. How does Python handle variables with no initial value?
In Python, variables can be declared without an initial value. These variables are assigned the value of “None”, which is a built-in data type that represents the absence of a value. The data type of the variable is determined at runtime based on the value that is assigned to it later in the code.
Q. How can developers debug errors related to dynamic typing in Python?
Debugging errors related to dynamic typing in Python can be more challenging than with statically typed languages. However, there are some strategies that developers can use to debug these errors, including:
- Using Python’s built-in “type” function to check the data type of a variable at various points in the code.
- Adding print statements to output the values of variables and their data types at various points in the code.
- Using Python’s built-in debugger to step through the code and track the values and data types of variables.
- Writing unit tests that cover different scenarios and data types to catch errors before they occur.
Q. What are some common use cases for dynamic typing in Python?
Dynamic typing in Python can be useful in a variety of scenarios, including:
- Working with data of varying types and formats, such as in data analysis or machine learning applications.
- Writing code that needs to be adaptable to changing inputs and scenarios, such as in web applications or scientific simulations.
- Creating prototypes or proof-of-concept applications where flexibility and speed of development are more important than strict data type checking.
Q. How does Python’s garbage collection work with dynamic typing?
Python’s garbage collector is responsible for freeing up memory that is no longer needed by the program. With dynamic typing, the garbage collector must track the data type of each variable and ensure that the appropriate memory is freed up when a variable’s value is no longer needed. This process is handled automatically by the interpreter and is transparent to the developer.
Q. How does dynamic typing impact Python’s performance?
Dynamic typing can have a small performance overhead in Python, as the interpreter needs to determine the data type of each variable at runtime. However, the impact of dynamic typing on performance is generally negligible for most applications, and the flexibility and adaptability that it provides can outweigh any potential performance costs.
I hope these additional questions and answers are helpful to you! Let me know if you have any further questions or if there is anything else I can assist you with.
Q. How does Python handle type checking with dynamic typing?
Python provides several built-in functions and modules that can be used to perform type checking and ensure that variables have the expected data types. These include the “isinstance” function, which checks if a variable is an instance of a specific data type, and the “typing” module, which provides annotations for function arguments and return types.
Q. How does dynamic typing impact code readability in Python?
Dynamic typing can have both positive and negative impacts on code readability in Python. On one hand, it can make code more concise and flexible, since developers don’t need to explicitly declare data types for each variable. On the other hand, it can make code more difficult to read and understand, since the data type of a variable may not be immediately clear from the code itself.
To improve code readability when working with dynamic typing, it’s important to use clear and descriptive variable names, add comments and documentation where necessary, and follow best practices for code organization and style.
Q. How does dynamic typing impact memory usage in Python?
Dynamic typing can impact memory usage in Python, since variables can have different data types and sizes throughout the program. This can result in more memory being used to store variables than in a statically typed language where variables have a fixed size and data type.
However, Python’s garbage collector helps to manage memory usage and ensure that memory is freed up when it’s no longer needed. Additionally, developers can take steps to minimize memory usage in their code, such as by using built-in data structures and being mindful of how data is stored and manipulated.
Q. How does dynamic typing impact Python’s performance when compared to statically typed languages?
Dynamic typing can result in some performance overhead in Python, since the interpreter needs to determine the data type of each variable at runtime. However, this overhead is generally small and is often outweighed by the benefits of dynamic typing, such as increased flexibility and adaptability.
When compared to statically typed languages, Python may have slightly lower performance in some scenarios, particularly in cases where strict data type checking is important. However, Python’s ease of use, flexibility, and large library of third-party modules make it a popular choice for a wide range of applications.
Q. What are some best practices for working with dynamic typing in Python?
Some best practices for working with dynamic typing in Python include:
- Using clear and descriptive variable names
- Adding comments and documentation where necessary
- Writing unit tests to catch errors related to data types
- Following Python’s style guide, PEP 8, to ensure consistent and readable code
- Using built-in data structures and functions where possible to minimize memory usage and improve performance.
arithmetic operators basics of python binary CBSE cbse class 12 cbse computer science class 12 cbse python revised syllabus 2021 class – 11 classs 11 computer science Computer science – PYTHON computer science python syllabus Conditional and Iterative statements Cookies and Tracking Scripts free python free python tutorial How to learn python programming How to learn python programming at home How to learn python programming FREE How to learn python programming STEP BY STEP Identity Protection mathematical operators Mohammad Amees Operators in Python Private browsing python Python Basics : The easiest way Python Data Types python free tutorial Python functions python notes python tutorial qissba qissba technologies qissba technology relational operators The If-Else statement The If-Statement in Python types of binary operators types of operators unary variable and assignment Virus Security Websites Track you What is Cyber Safety
Read more: Dynamic Typing in Python CBSE – Class 12