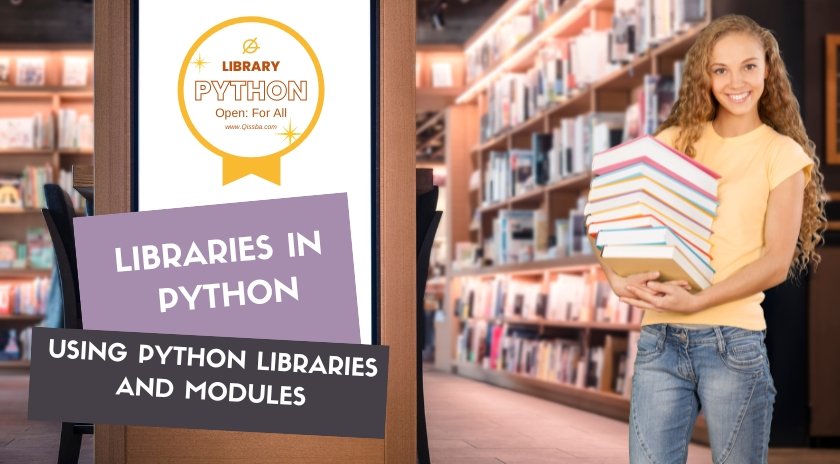
Creating Libraries in Python | Using Python Libraries and Modules
In this tutorial, “ Creating Libraries in Python: Using Python Libraries and Modules”, we will provide a comprehensive introduction to Basics of Libraries and Modules in python Programming including
- Introduction to String in Python.
- Libraries – Introduction
- Libraries – What is a Library
- Libraries – What is a module?
- Libraries – Importing Modules in a Python Program
- Libraries – Using Python Standard Library’s Functions and Modules
- Libraries – Creating a Python Library
And, by the end of this tutorial, readers will have a solid understanding of all Basics of string in Python and will be able to use this knowledge in their own programming projects.
Also this tutorial covers all necessary topics/concepts required to complete your exams preparations in CBSE schools / classes.
Python Libraries – An Introduction
You all must have read and enjoyed a lot of books – story, novels, course-books, reference books etc. and, if you recall, all these books’ types have one thing in common. Confused? Don’t be – all these books’ types are further divided into chapters. Can you tell, why is this done? yeah, you are right. Putting all the page of one or novel book together, with no chapters, will make the book boring and difficult to comprehend. so, dividing a bigger unit into smaller manageable units is a good strategy.
Similarly, in programming, if we create smaller handleable units, these are called modules. A related term is library here. A library refers to a collection of modules. That together cater to specific type of need or applications e.g., NumPy library of python caters to computing needs. In this chapter, we shall talk about using some python libraries as well as creating own libraries/modules.
What is a Library
In this tutorial, we have a short Introduction to Python Libraries. Before starting few words from your teacher are as :-
As mentioned in introduction that a library is a collection of modules (and packages) that together cater to specific type of application or requirements. A library can have multiple modules in it. This will become clearer to you when we talk about ‘what module are’ in coming lines.
Some commonly used python libraries are as listen below:
Python standard library
This library is distributed with python that contains modules for various type of functionalities. Some commonly used n=modules of python standard library are:
- Math module, which provides mathematical function to support different types of calculation.
- cmathmodule, which provides mathematical function for complex numbers.
- Random module, which provides function for generating pseudo-random numbers.
- Statistics module, which provides mathematical statistics functions.
- Urllib module, which provides URL handling function so that you can access websites from within your program.
NumPy:
This library provides some advance math functionalities along to create and manipulate numeric arrays.
SciPy
This another useful library traditional python user interface tool for scientific calculations.
tkinter
This library provides traditional python user interface toolkit and helps you to create user-friendly GUI interface for different types of applications.
Matplotlib
This library provides offers many functions and tools to produce quality output in variety of formats such as plots, charts, graphs etc.
A library can have multiple modules in it. Let us explore, what a module means.
What is a module?
The act of portioning a program into individual components (known as modules) is called modularity. A module is a separate unit in itself. the justification for portioning a program is that
- It reduces its complexity to some degree and
- It creates a number of well-defined, documented boundaries within the program.
Another useful feature of having modules, is that its contents can be reused in other programs, without having to rewrite or recreate them.
For example, if someone has created a module, is that its contents can be reused in other programs, without having to rewrite or recreate them.
For example, if someone has created a module say ‘play audio’ to play different audio format, coming from different sources, e.g., mp3player, FM-radio player, DVD player etc. now, while writing a different program, is someone wants to incorporate FM-radio into it, he need not re-write the code for it. Rather, he can use the FM-radio functionality from play audio module. Isn’t that amazing? re-usage without any re-work – well, that’s the beauty of modules.
Structure of a Python Module
A python module can contain much more than just function. A python module is anormal python file (. py file) containing one or more of the following objects related to a particular task:
So, we can say that the module ‘XYZ’ means it is file ‘XYZ.py’.
Python comes loaded with some predefined module that you can use and you can even create your own modules. The python modules that come preloaded with python are called standard library modules. You have worked with one standard library module math earlier and in this tutorial, you shall also learn wo work with another standard library module: random module.
Figure shows the general composition / structure of a python module.
Let’s have a look at an example module. Namely temp conversion to figure below.
(Please note, it is not form python’s standard library: it is a user created module, shown here for your understanding purposes).
The elements of module shown in figure are:
The docstrings of a module are displayed as documentation, when you issue following command on python’s shell prompt >>>, after importing the module with import<module-name>command:
Help (module-name >)
For example, after importing module given in fig 4.2, i.e., module tempconversion, if we write
Help(tempconversion)
Python will display all docstrings along with module name, filename, functions’ name and constant as shown below:
>>>import tempconversion
>>>help(tempconversion)
Help on module temp conversion:
There is one more function dir.() when applied to a module gives you names of all that is defined inside the module.(see below)
>>>import tempconversion
>>>dir(tempconversion)
[‘FREEZING_C’, ‘FREEZING_F’, ‘_built-ins_’ ,’_doc_’,’_file_’,_name_’,’_package_.,to_centigrade’,’to_fahrenhelt’]
General docstring conventions are:
- first letter of first line is a capital letter.
- Second line is blank line.
- Rest of the details begin from third line.
Importing Modules in a Python Program
As mentioned before, in Python if you want to use the definition inside a module, then you need to first import the module in your program. Python provides import statements to import modules in a program. The import statement can be used in two forms:
- To import entire module: the import <module> command
- to import selected objects: the from <module> import>object> command from a module
Following subsections will make the utility of both these import commands clear.
importing entire module
The import statement can be used to import entire module and even for importing selected items. To import entire module, the in-import statement can be used as per following syntax: (please remember, in syntax, [] specify optional elements.)
The import statement internally executes the code of module file and then makes it available to your program. The import statement like the one shown above, imports entire module i.e., everything defined inside the module – function definitions, variables, constants etc.
After importing a module, you can use any function/definition of the imported module as per following syntax:
<module-name>,<function-name>()
This way of referring to a module’s objects is Calles dot notation. For example, consider the module tempConversion given in figure 4.2 To use its function to centigrade(), we’II be writing :
The name of a module is stored inside a constant _name_ (prefix & suffix are having two underscores). You can use it like :
Import time
print(time._name_)
It will print the name of imported module (see below)
>>> import time
>>> print time._name_
time
>>> |
You can given alias name to imported module as :
Import<module> as <aliasname>
example
import tempConversion as tc
Now you can use name tc for the imported module e.g., tc.to_ centigrade().
importing select objects from a module
If you want to import some selected items, not all from a module, then you can use from <module> import statement as per following syntax:
from <module> import <objectname> [, <objectname> [ …] |*
To import Single Objects
If you want to import a single object from the module like this so that you don’t have to prefix the module’s name, you can write the name of object after keyword import. For instance, to import just the constant pi from module math, you can write:
from math import pi
Now, the constant pi can be used and you need, not prefix it with module name. That is, to print the value of pi, after importing like above, you’II be writing:
Do not use module name with imported object if imported through from < module>import command because now the imported objects is part of your program’s environment.
To Import Multiple Objects
If you want to import multiple objects from the module like this so that you don’t have to prefix the module’s name, you can write the comma separated list of objects after keyword import. For instance, to import just two functions sqrt() and pow() from math module, you will write :
from math import sqrt, pow
To Import All Object of a Module
If you want to import all the items from the module like this so that you don’t have to prefix the module’s name, you can write :
from<module>import*
That is, to import all the items from module math, you can write :
from math import*
Now you can use all the defined functions variables etc. from math module, without having to prefix module’s name to the imported item name.
Python’s Processing of import < module > command
With every import command issued, python internally does a series of steps. In this section, we are briefly discussing the same. Before we discuss the internal processing of import statements, let’s first discuss namespace – an important and related concept, which plays a role in internal processing of import statement.
Name space
A namespace, in general, is a space that holds a bunch of names. Before we go to explain namespaces in python terms, consider this real life example.
From the above real – life example, we can say that Kerala ward has its own namespace where there no two names as Nalini; same holds for Delhi and Odisha.
In python terms, namespace can be thought of as a named environment holding logical grouping of related objects. You can think of it as named list of some names.
For every module (. Py file), python creates a namespace having its name similar to that of module’s name. That is, the name of module time’s namespace is also time.
When two namespace come together, to resolve any kind of object – name dispute, python asks you to qualify the names of objects as <module-name>.<object- name>,just like in teal life example, we did by calling Delhi’ s Nalini or Odisha’ s Nalini and so on. Within a namespace, an object is referred without any prefix.
Processing of import < module > command
When you issue import < module> command, internally following things take place:
- The code of imported module is interpreted and executed.
- Defined functions and variables created in the nodule are now available to the program that imported module.
- For imported module, a new namespace is setup with the same name as that of the module.
For instance, you imported module my Mod in your program. Now all the objects of module my Mod would be referred as my mod. <object- name>, e.g., if my Mod has a function defined as checksum ( ), then it would be referred to as my mod. Checksum ( ) In your program
Processing of from <module> import <object> command
When you issue from <module>import <object> command, internally following things take place:
- The code of imported module is interpreted and executed 4.
- Only the asked functions and variables from the module are made available to the program.
- No new namespace is crated, the imported definition is just added in the current namespace
This Figure illustrates this difference.
That means if your program already has a variable with the same name as the one imported via module, then the variable in your program will hide imported member with same name because there cannot be two variables with the same name in one namespace
Following code fragment illustrates this.
Let’s consider the module given in above figure
from tempconversion import *
FREEZING _ C = – 17. 5
#It will hide FREEZING _ C of tempconversion module
print (FREEZING _ C)
The above code will print
-17. 5
If you change above code to the following (we made FREEZING _ C = – 17.5 as a COMMENT, see below:
from tempconversion import *
# FREEZING _ C = -17.5 # it is just a comment now print (FREEZING _ C)
Now the above code will give result as:
0.0
As no variable from the program shares its name, hence it is not hidden.
Sometimes a module stored inside another module Such a sub module can also be imported as:
from<parent module> import <sub module> [as <alias name>]
Example
from product import views
from product import views as PV
Where PV is alias name for product views (sub module)
Using Python Standard Library’s Functions and Modules
Python’s standard library is very extensive that offers many built- in functions that you can use without having to import any library. Python’s standard library is by default available, so you don’t need to import it separately.
Python’ s standard library also offers, other that the built- in functions, some modules for specialized type of functionality, such as math module for mathematical functions; random module for pseudo – random number generation; urllib for functionality for adding and using web sites’ address from within program; etc.
In this section, we shall discuss how you can use pythons built – in function and import and use various modules and python’s standard library.
using python’s built-in functions
The python interpreter has a number of functions built into it that are always available; you need not import any module for them. In other words, the built in functions are part of current namespace of python interpreter. So you use built-in functions of python directly as:
<function- name> ( )
For example, the functions that you have worked with up till now such as input (), int (), float (), type (), Len () etc. are all built in functions, that is why you never prefixed them with any module name.
python’s built-in mathematical functions
Python provides many mathematical built-in functions. You have worked with many built-in mathematical functions of python in your previous class. Let us quickly recall those and then we shall talk about some more such functions.
Let us now talk about some more built in mathematical functions.
Table 4.1 some useful built-in mathematical functions
Let us now talk about some more built-in mathematical functions.
some useful built-in mathematical functions.
Consider following program that uses two more built-in functions:
Python code example:
Write a program that inputs a real number and coverts it to nearest integer using two different built-in functions. It also displays the given number rounded off to 3 places after decimal.
num = float (input (“ Enter a real number : ” ) )
thum = int (num)
rrum = round (num)
print (“Number” , num, “converted to integer in 2 ways as” , thum, “and” , rrum )
rrum 2 = round (num, 3)
print (num, “rounded off to 3 places after decimal is” , rrum2 )
Sample run of above program is:
Enter a real number: 5. 555682
Number 5. 555682 converted to integer in 2ways as 5 and 6
5.555682 rounded off to 3 places after decimal is 5.556
** The behavior of round ( ) can be surprising for floats, e.g., round (0.5) and round (-0.5) are 0, and round (1.5) is 2.
python’s built-in string functions
Let us now use some string functions. Although you have worked with many string functions in your previous class, let us use three new string based functions. These are:
- <str>. Join (<string iterable>) – joins a string or character (i.e., <str>) after each member of the string iterator i.e., a string based sequence.
- <str>. Split(string / char> ) – splits a string (i.e., <str> ) based on given string or character (i.e., <string / char>) and returns a list containing split as members.
- <str>. Replace(<word to be replaced>, <replace word> ) – replaces a word or part of the string with another in he given string <str>.
Let us understand and use these string functions practically. Carefully go through the examples of these as given below:
(str>. Join ( )
- If the string based iterator is a string then the <str> is inserted after every character of the string, e.g.,
>>>” *” . join (“Hello” ) see, a character is joined with each member of
‘H*e*l*l*0’ the given string to form the new string
>>>”***” .join (“TRIAL”) see, a string (“***” here) is joined with each
‘T***R***I***A***L’ member of the given string to form the new
2. If the string based iterator is a list or tuple of strings then, the given string / character is joined with each member of the list or, BUT the tuple or list must have all member as strings otherwise python will an error.
<str >. Split ( )
- If you do not provide any argument to split then by default it will split the given string considering whitespace as a separator, e.g.,
2. If you provide a string or a character as an argument to split ( ), then the given string is divided into parts considering the given string / character as separator character is not included in the split strings e.g.,
<str>. replace ()
Python code example:
Write a program that inputs a main string and then creates an encrypted string by embedding a short symbol-based string after each character. The program should also be able to produce the decrypted string from encrypted string.
def encrypt (sttr, enkey ):
return enkey. Join (sttr)def decrypt (sttr, enkey):
return sttr. Split (enkey)#-main –
main string = input (“Enter main string :”)
encrypts = input (“Enter encryption key: ”)
enstr = encrypt (main string, encryptstr)
deLst = decrypt (enstr, encryptstr)
# dolts is the form of a list , converting it to string below
destr = “ “ . Join (delist)
print (“the encrypted string is”, enstr)
print (“string after decryption is:”, destr)
The sample run of the above program is as shown below:
Enter main string: My main string
Enter encryption key: @$
The encrypted string is m@$y@$ @$m@$a@$i@$n@$ @$s@$t@$r@$i@$n@$g
string after decryption is : My main string
working with some standard library modulus
Other than built-in functions, standard library also provides some modules having functionality for specialized actions. Let us learn to use some such modules. In the following lines we shall talk about how to use some useful functions of random and string modules6 of Python’s standard library.
using random module
Python has a module namely random that provides random-number7 generations. A random number in simple words means-a number generated by chance, i.e., randomly.
To use random number generators in your Python program, you first need to import module random using any import command example:
import random
Some most common random number generator functions in random module are:
Let us consider some examples. In the following lines we are giving some sample codes along with their output.
2. To generate a random floating-point number between range lower to upper using random ():
- Multiply random () with difference of upper limit with lower limit, i.e., (upper-lower)
- Add to it lower limit
For example, to generate between 15 to 35, you may write:
3. To generate a random integer number in range 15 to 35 using ran dint(), write:
>>>print (random. ran dint (15,35))
16 The output generated is integer between range 15 to 35
4. To generate a floating-point random number in the ranges11…55 or 111…55, after importing random module using import statement, you may write:
>>>random. Uniform (11,55)
output : 41.3458981317353
>>>random. Uniform (111,55)
output : 60.03906551659219
5. To generate a random integer in the ranges 23.47 with a step 3 or 0.235, after importing random module using import statement, you may write:
>>> random. Rand range (23, 47,3)
output : 38
>>> random. Rand range (235)
output : 126
Python Code Example:1
Given the following Python code, which is repeated four times. What could be the possible set of outputs out of given four sets (ddd represent any combination of digits)?
import random
print (15 + random. Random () *5)
dddd, 19. dddd, 20. dddd, 15. dddd
dddd, 17. dddd, 19. dddd, 18. dddd
dddd, 16. dddd, 18. dddd, 20. dddd
dddd,15. dddd,15. dddd, 15. dddd
Solution:
Option (ii) and(iv) are the correct possible outputs because:
- Random () generates number N between range 0.0<= N <1.0
- When it is multiplied with 5, the range becomes 0.0 to <5
- When 15 is added to it, the range becomes 15 to <20
Only option (iii) and (iv) fulfill the condition of range 15 to < 20.
Python Code Example: 2
What could be the minimum possible and maximum possible numbers by following code?
import random
print (random. ran dint (3,10)-3)
Solution:
Minimum possible number = 0
Maximum possible number = 7
Because,
- Ran dint (3,10) would generate a random integer in the range 3 to 10
- Subtracting 3 from it would change the range to 0 to 7 (because if ran dint (3, 10) generates 10 then -3 would make it 7; similarly, for lowest generated value 3, it will make it 0)
Python Code Example: 3
In a school fest, three randomly chosen students out of 100 students (having roll numbers1-100) have to present bouquets to the guests. Help the school authorities choose three students randomly.
Solution:
import random
Student1 = random. ran dint (1,100)
Student2 = random. ran dint (1,100)
Student3 = random. ran dint (1,100)
print (“3 chosen students are’’,)
print (student1, student2, student3)
using string module:
Python has a module by the name string that comes with many constants and classes. It also offers a utility function cap word (). Let us talk about some useful constants defined in the string module.
Please note that like other modules, before you can use any of the constant/functions defined in the string module, you must import it using an import statement:
import string
Some useful constants defined in the string module are being listed below:
The string module also offers a utility function cap word ():
You can obtain the value of the constants defined in string module by simply giving their name with the string module name after importing string module, e.g.,
>>import string
>>>string. ascii_letters
‘abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ’
>>> STRING.DIGITS
‘0123456789’
>>> string. ascii_uppercase
‘ABCDEFGHIJKLMNOPQRSTUVWXYZ’
>>> string punctuation
‘!’’#$%&’()*+,-./:;?@[\]^_’{|}~’
You can use cap words () using the string module name and passing the string name as its argument,
example:
>>> import string
>>> line = ‘’this is a simple line\n new line’’
>>> string. cap words (line)
Creating a Python Library
Other than standard library, there are numerous libraries available which you can install and use in your programs some such libraries are NumPy, SciPy, tkinter etc.
One of these libraries NumPy, has been covered briefly
another library – tkinter. You can also create your own libraries.
You have been using terms modules and libraries so far. Let us talk about a related word. Package in fact most of the times library and package terms are used interchangeable.
A package is collection of python modules under a common namespace, created by placing different modulus on a single directory along with some special files (such as_init_py)
In a directory structure, in order for a folder (containing different modules i.e., p.y. files) to be recognized as a package a special file namely_init_py must also be stored in the folder, even if the file_init_py is empty.
A library can have one or more packages and sub packages.
Let us now learn how you can create your library in python.
Now on, we shall be using word package as we shall be creating simple libraries that can be called package interchangeable.
structure of a package
As you know that python packages are basically collection of modules under common namespace. This common namespace is created via a directory that contains all related modules. But here you should know one thing that NOT ALL folders having multiple.py. files (i.e., modules) are packages in order for a folder contain python files it be recognized as a package, an _init_py file (even if empty) must be part of the folder. Following figure (fig4.4) explain it.
Procedure for Creating Packages
In order to create a package, you need to follow a certain procedure as discussed below. Major steps to create a package are:
Decide about the basic structure of your package.
It means that you should have a clear idea about what will be your package’s name (i.e., a directory/folder with that name will be created) and what that all modulus and subfolder (to serve as sub packages) will be part of it.
(Just keep in mind that while naming the folder/subfolder; don’t use another underscore – separated don’t use any other word separators, at all (not even hyphens)) for instance, we are going to create the packages shown on the right.
Create the directory structure having folders with names of packages and sub packages.
In our example shown above, we created a folder by the name Librcomputersubjects.
Inside this folder, we created files/modules, i.e., the. Py files and sub folders with their own. py files. Now out topmost folder has the packages name as per above figure and subfolder have name as the sub packages (as per adjacent Figure).
But this is not yet eligible to be a package in python as there is no _init_py file in the folders (s).
Create _init_py files in packages and sub packages folders.
We created and empty file and saved it as’’ _init_py’’ and then copied this empty _init_py file to the package and sub package.
Now our directory structure looked like the one shown here.
Without the _init_py file, python will not look submodules inside that directory, so attempts to import the module will fail.
Associate it with python installation.
Once you have your package directory ready. You can associate it with python by attaching it to python’s site packages folder of current python distribution in your computer. You can import a library and package in python only if it is attached to his site packages_ folder.
- In order to check the path of the site packages folder of python on the python prompt, type the following two commands one after another and try to locate the path of site-packages folder:
The sys.path attribute gives an important information about PYTHONPATH, which specifies the directories that the python interpreter will look in when importing modules.
If you carefully look at above result of print (sys. path) command you will find that the first entry in PYTHONPATH is ‘‘, it means that the python interpreter will first look in the current directory when trying to do an important if the asked module/packages is not found in current directory. Then it check the directory listed in PYTHONPATH.
- Once you have figured out the path of site-packages folder, simplest way is to copy your own package-folder (e.g., Librocomputersubject that we created above) and paste it in the folder. (But this is not the standard way.)
On our window installation, we simply opened the site-packages folder as per above path and pasted the complete Librcomputersubjects folder there.
Final
After copying your package folder in site-packages folder of your current python installation, now it has become a python library so that now you can import its modules and use its functions.
using/importing python libraries
Once you have created your own package (and sub package) and attached it to site-packages folder of current python installation on your computer, you can use them just as the way you use other python libraries.
To use an installed python library, you need to do the following:
- Import the library using import command:
Import <full name of library >
- Use the function, attributes etc. Defined in the library by giving their full name.
For instance, to use package Librcomputerpackages’s two module files, which are shown here, you can import them and then use them as shown below:
As you can see that the details module has a function namely Subjects List () and CSXI modules has two functions namely syllabus () and about ().
- To import module details, you can write import command as:
Import LibreComputerSubject.detaols
And use its functions by giving its full name i.e.
Import LibreComputerSubject.detaols,<function>()
- To import module CSXL, you can write command as:
import LibreComputerSubjects.ComputerScience.CSXI
And use its functions by giving its full name i.e.
LibreComputerSubjects.ComputerScience.CSXI,<function>()
We have kept this discussion very simple and basic. You can even create installation libraries in python. The installable libraries also have a setup.py file. But we are not going into those details as this is beyond the scope of this book.
However, we shall recommend one thing: while naming a library, try to use all lowercase letter although we used capital letters in above example (Librcomputersubjects). we did this to make it more readable so that you could understand it easily. But if you are creating an actual library and you want it to be used by any python user then it try to give it unique name (i.e., check in PYPI8-python package index if your library name is unique) all in lowercase so that everyone can use it without bothering about the case of the letters.