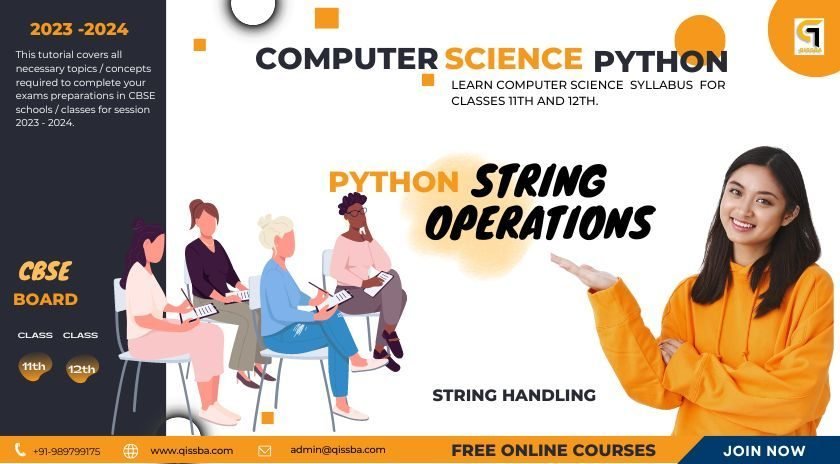
String Operations in Python: String Handling | CBSE – Class 12
In this section, “String Operations in Python: String Handling Best Practices”, we will provide a comprehensive introduction to Python String Operation with their Operators including
- List of String Operators in Python
- Understanding String Operations in Python
- Best Practices for String Handling in Python
And, by the end of this tutorial, readers will have a solid understanding of String operators and their operations in Python and will be able to use this knowledge in their own programming projects.
Also this tutorial covers all necessary topics/concepts required to complete your exams preparations in CBSE schools / classes.
Introduction:
Python is a powerful and versatile programming language that is commonly used in various domains such as web development, data science, and automation.
One of the key strengths of Python is its ability to handle strings with ease, making it a popular choice for text-based applications.
In this article, we will explore some of the most important string operations in Python and provide best practices for handling strings in your code.
List of String Operators in Python:
Python allows several string operators that can be applied on the python string are as below:
- Assignment operator: “=.”
- Concatenate operator: “+.”
- Escape sequence operator: “\.”
- Membership operator: “in” & “not in”
- Comparison operator: “==” & “!=”
- Formatting operator: “%” & “{}”
- Repetition operator: “*.”
- Slicing operator: “[]”
Understanding String Operations in Python:
Strings are a fundamental data type in Python, and a good understanding of string operations is essential for efficient programming. The following are some of the most important string operations in Python:
Concatenation:
The concatenation operation allows you to combine two or more strings together using the ‘+’ operator. For example, ‘Hello’ + ‘ World’ would result in ‘Hello World’.
Indexing:
Strings in Python are indexed, which means that each character in a string is assigned a unique position or index number. You can access individual characters in a string using their index number. For example, ‘Python'[0] would return ‘P’.
Slicing:
Slicing is the process of extracting a substring from a larger string. You can slice a string using the ‘:’ operator. For example, ‘Python'[0:3] would return ‘Pyt’.
Formatting:
String formatting allows you to insert variables and other dynamic content into a string. Python provides several ways to format strings, including the ‘%’ operator, the ‘format()’ method, and f-strings.
Best Practices for String Handling in Python:
Now that we understand the various string operators in Python, let’s discuss some best practices for handling strings in your code.
Use Raw Strings for Regular Expressions:
Regular expressions are a powerful tool for string manipulation in Python. To make regular expressions more readable, use raw strings by prefixing the regular expression with the letter ‘r’. For example, r’\d{3}-\d{2}-\d{4}’ would match a string in the format of a social security number.
Use String Formatting Instead of Concatenation:
While concatenation is a useful string operation, it can be cumbersome when you need to combine a large number of strings. Instead, consider using string formatting, which allows you to insert values into a string using placeholders.
Avoid Mutable Strings:
Strings in Python are immutable, which means they cannot be modified once they are created. Therefore, avoid using mutable strings like lists or arrays, which can slow down your code and lead to unexpected results.
Use the Join Method for String Concatenation:
The join() method is an efficient way to concatenate a large number of strings. It works by joining a list of strings together using a specified delimiter. For example, ‘-‘.join([‘1’, ‘2’, ‘3’]) would result in ‘1-2-3’.
Conclusion:
String operations are an essential part of Python programming, and a good understanding of best practices for handling strings can make your code more efficient, readable and maintainable. In this article, we have discussed some of the most important string operations in Python, and provided best practices for handling strings in your code. Remember to use the focus keyword “string operations in python” in a natural and organic way throughout your content, while prioritizing readability and user engagement.