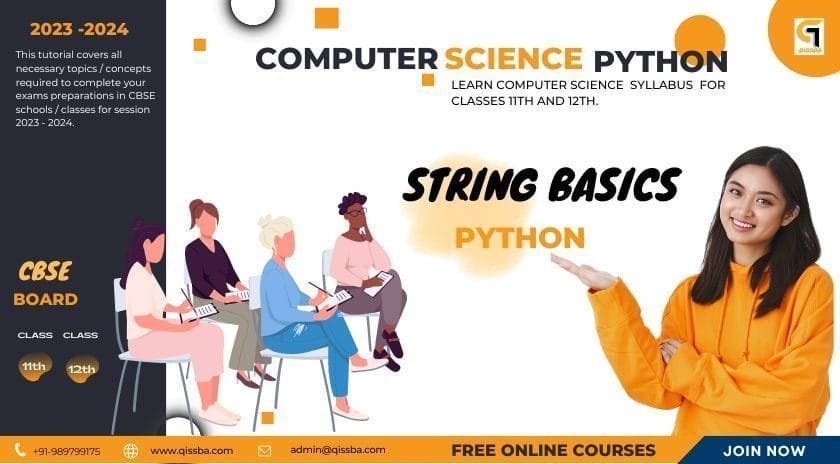
Python String Basics: An Introduction | CBSE – Class 12
In this section, “Python String Basics: An Introduction to Working with Text Data”, we will provide a comprehensive introduction to Basics of strings in python, including
- What are Strings in Python.
- Creating Strings in Python.
- Accessing Characters in a String.
- String Concatenation.
- Advantages – Importance of strings in python
And, by the end of this tutorial, readers will have a solid understanding of Basics string in Python and will be able to use this knowledge in their own programming projects.
Also this tutorial covers all necessary topics/concepts required to complete your exams preparations in CBSE schools / classes.
Introduction: Python text data basics
Python is a powerful programming language used in many fields, including data analysis and web development. One of the most fundamental data types in Python is the string. Strings are a collection of characters, and they are used to represent text data in Python. In this blog post,
we will provide an introduction to working with text data in Python, specifically focusing on strings in Python.
What are Strings in Python?:
Strings are a sequence of characters enclosed in single or double quotes. They can be used to represent text data, including words, sentences, and even entire documents. Strings are immutable, which means that once a string is created, it cannot be modified. However, you can create a new string by concatenating two or more strings.
Creating Strings in Python:
To create a string in Python, you simply need to enclose a sequence of characters in single or double quotes. For example:
my_string = "Hello, world!"
And, you can also use triple quotes to create a string that spans multiple lines:
my_string = """This is a multi-line string"""
Accessing Characters in a String:
You can access individual characters in a string using indexing. In Python, indexing starts at 0, which means that the first character in a string has an index of 0.
For example:
my_string = "Hello, world!" print(my_string[0])
# Output: H
You can also access a range of characters in a string using slicing. Slicing allows you to extract a substring from a string. For example:
my_string = "Hello, world!" print(my_string[0:5])
# Output: Hello
String Concatenation:
You can concatenate two or more strings using the + operator. For example:
first_name = "John" last_name = "Doe"
full_name = first_name + " " + last_name print(full_name)
# Output: John Doe
Example of String in Python:
Now, a python code example for showing some basic operations on python string like
- Accessing characters of a python string
- Slicing of python string
- Concatenation of python string
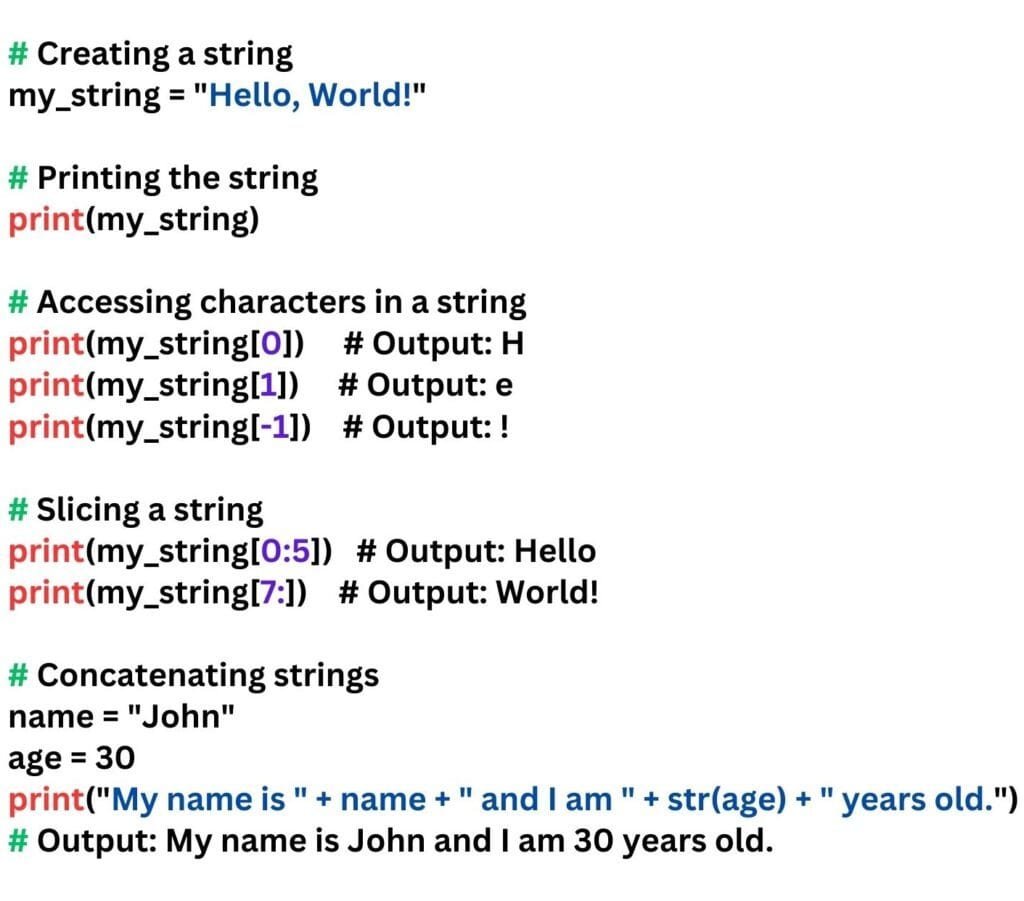
In this example, we create a string my_string
that contains the text “Hello, World!”.
and demonstrate, how to access individual characters in the string using indexing, with my_string[0]
returning the first character “H”.
Then, we use slicing to extract a substring from the original string, with my_string[0:5]
returning the first five characters “Hello”.
We then show how to concatenate strings using the +
operator, with the example concatenating the variables name
and age
with a string to create the output “My name is John and I am 30 years old.“.
Note that we use the str()
function to convert the integer age
to a string before concatenation.
Basic String Methods in Python:
Python provides a wide range of built-in string methods that can be used to manipulate strings. Here are a few common string methods:
- upper(): Converts all characters in a string to uppercase.
- lower(): Converts all characters in a string to lowercase.
- strip(): Removes any whitespace from the beginning and end of a string.
- replace(): Replaces a specified substring with another substring.
Here is an example of using these methods:
String Method upper() Example:
my_string = " Hello, World! "
print(my_string.upper())
# Output: HELLO, WORLD!
String Method lower() Example:
my_string = " Hello, World! "
print(my_string.lower())
# Output: hello, world!
String Method strip() Example:
my_string = " Hello, World! "
print(my_string.strip())
# Output: Hello, World!
String Method replace() Example:
my_string = " Hello, World! "
print(my_string.replace("Hello", "Hi"))
# Output: Hi, World!
Advantages – Importance of strings in python:
Strings are an integral part of programming, and they play a crucial role in data representation, user input handling, web development, natural language processing, debugging, and testing. Understanding the importance of strings in programming is essential for any developer, data analyst, or scientist working with text data. In this post, Here is list to explore the importance of strings in programming and how they are used in various applications.
- Data Representation:
- User Input Handling:
- Web Development:
- Natural Language Processing:
- Debugging and Testing:
Conclusion:
Now, you have basic knowledge about python strings like
- That python strings are characters enclosed in quotes of a type – single quotation marks, double quotation marks and triple quotation marks.
- An empty string is a string that has 0 characters (i.e., it is just a pair of quotation marks) and that python strings are immutable.
- Strings are sequences of characters, where each character has a unique position-id/index. The indexes of a string begin from 0 to length -1 ) in forward direction and -1, -2, -3, …., – length in backward direction.
In this blog post, we have provided an introduction to working with text data in Python, specifically focusing on strings in Python. We covered how to create strings, access characters in a string, concatenate strings, and use built-in string methods. By mastering these basics, you will be well on your way to working with text data in Python.