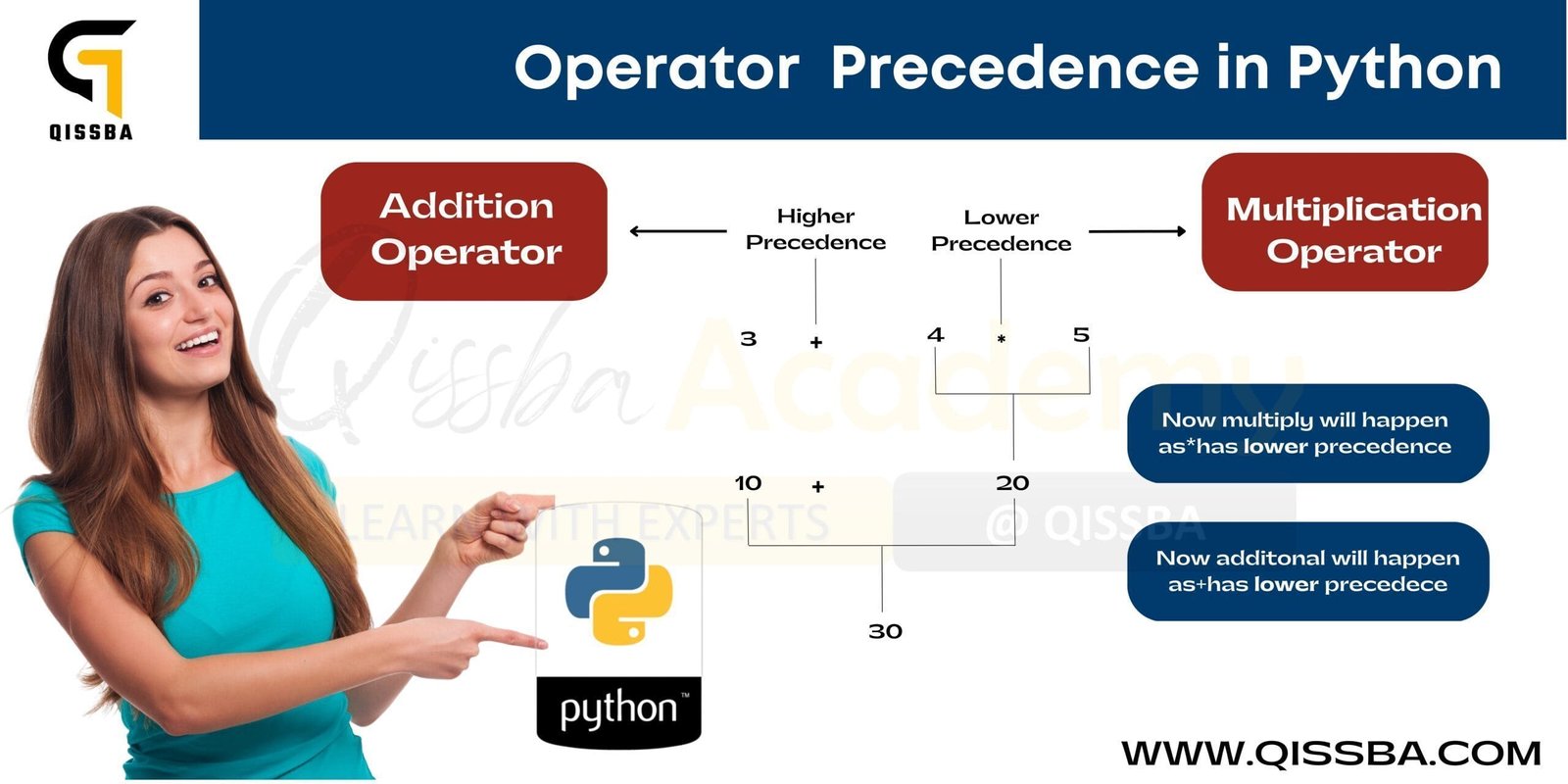
Precedence of Operators in Python | CBSE class 11
Dear Class 11th STUDENTS,
Welcome to this tutorial of Unit-2 from your CBSE Syllabus of Computer Science.
In this tutorial, we shall be learning our chapter-2 from Unit 2: Programming and Computational Thinking (PCT-1) as CBSE BOARD suggested to learn about computer system and its organisation to complete this section.
After going through this tutorial, you will be able to understand the following topics from your latest syllabus 2025-26.
Unit 2: Programming and Computational Thinking (PCT-1)
Chapter 5: Precedence of Operators in Python
- What is the Precedence of Operators in Python?.
- List of operator’s precedence in python.
- Python Operators Precedence Rule – PEMDAS.
- Examples of Precedence of Operators in Python.
- Importance of Precedence Order of Operator in Python.
- Best Practices for Using Precedence of Operators.
- Important Questions and Answers.
I advice to check the latest syllabus given by CBSE Board at its Official website: www.cbseacademic.nic.in
Also, in this tutorial we will covers all necessary topics/concepts required to complete your exams preparations in CBSE classes 11th.
Also, you can Sign Up our free Computer Science Courses for classes 11th and 12th.
NOTE:
- We are also giving some important Questions & Answers for better understanding as well as preparation for your examinations.
- You may also download PDF file of this tutorial from our SHOP for free.
- For your ease and more understanding, we are also giving the video explanation class of each and every topic individually, so that you may clear your topics and get success in your examinations.
Introduction:
In Python programming language, operators are used to perform mathematical, logical, and comparison operations. These operators are evaluated based on their precedence, which determines the order in which they are evaluated in an expression. Understanding the precedence of operators is crucial for writing correct and efficient Python code. In this article, we will discuss the precedence of operators in Python and provide examples to illustrate their use.
What is the Precedence of Operators in Python?
The precedence of operators in Python is a set of rules that determine the order in which operators are evaluated in an expression. Python follows a well-defined order of precedence for operators, where certain operators are evaluated before others. The order of precedence can be summarized as follows:
- Parentheses – highest precedence
- Exponentiation (**) – second highest precedence
- Multiplication, Division, and Modulo (%) – third highest precedence
- Addition and Subtraction – fourth highest precedence
- Bitwise Shifts – fifth highest precedence
- Bitwise AND (&) – sixth highest precedence
- Bitwise XOR (^) – seventh highest precedence
- Bitwise OR (|) – eighth highest precedence
- Comparison Operators – ninth highest precedence
- Boolean NOT – tenth highest precedence
- Boolean AND – eleventh highest precedence
- Boolean OR – lowest precedence
Using Parentheses to Control Precedence One of the most important ways to control the precedence of operators is through the use of parentheses. Parentheses are used to group sub-expressions together, and the expressions within parentheses are always evaluated first.
For example, the expression
(2 + 3) * 4
is evaluated differently than
2 + 3 * 4.
List of Operator’s Precedence in Python:
Here is a list of operator’s precedence from highest to lowest for the operator that python provides.
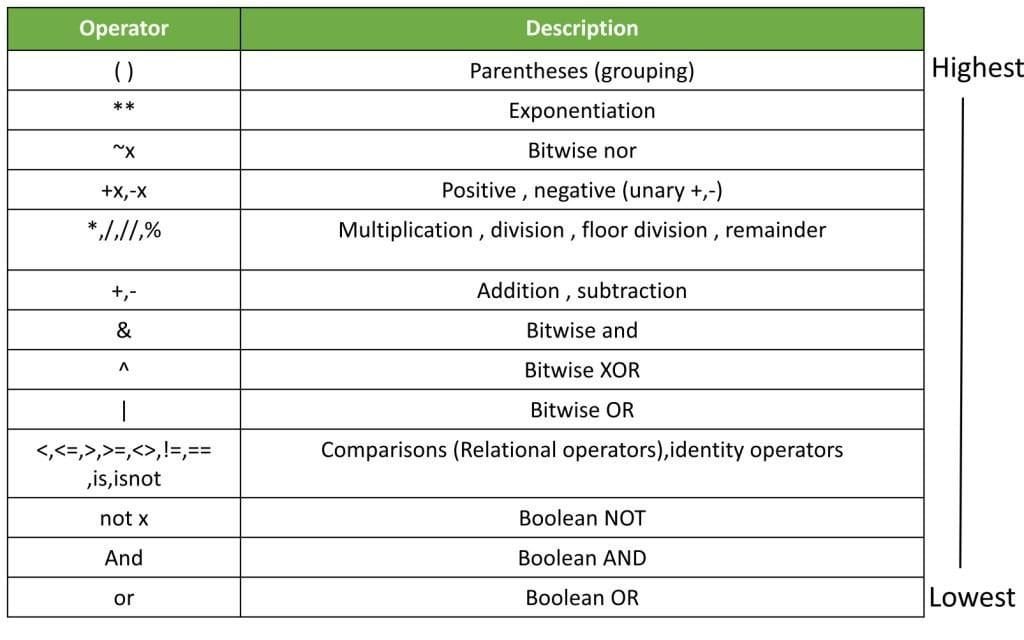
When an expression or statement involves multiple operators, python resolves the order of execution of operator precedence by following the above precedence orders in python.
This precedence order in similar to BODMAS rule of mathematics.
Example
consider the following example of given expression what will be the final output ?.
a,b=3,6
C=5
z=a*b/c//a
Now , you try to solve these expressions:
14 / 13 % 15
a % b // a,
where a=16 & b=15
Python Operators Precedence Rule – PEMDAS:
- P – Parentheses.
- E – Exponentiation.
- M – Multiplication.
- D – Division.
- A – Addition.
- S – Subtraction.
It’s important to keep the following rules in mind when working with operator precedence in Python:
- Operators with higher precedence are evaluated first.
- If two operators have the same precedence, they are evaluated from left to right.
- Parentheses can be used to group operations and override the default precedence.
Examples of Precedence of Operators in Python:
Let’s look at some examples to illustrate the precedence of operators in Python:
Example 1:
3 + 4 * 5
In this example, the multiplication operator (*) has higher precedence than the addition operator (+). Therefore, the expression is evaluated as
3 + (4 * 5) = 23.
Example 2:
10 % 3 * 2
In this example, the modulo operator (%) has higher precedence than the multiplication operator (*). Therefore, the expression is evaluated as
(10 % 3) * 2 = 2.
Python Bitwise Operator Precedence:
Python provides several bitwise operators for performing operations at the bit level. The following chart shows the order of precedence for Python bitwise operators:
- Bitwise NOT: ~x
- Bitwise AND: x & y
- Bitwise XOR: x ^ y
- Bitwise OR: x | y
- Bitwise Left Shift: x << y
- Bitwise Right Shift: x >> y
Precedence rules for operators in Python are the same for bitwise operators as well.
Why Precedence Order in Python?:
The Precedence Order of Operators in Python is important because it determines the order in which operators are evaluated in an expression. If the order of evaluation is not correct, it can lead to unexpected results and errors in the program. For example,
consider the expression
3 + 4 * 5.
If the addition operator (+) is evaluated before the multiplication operator (), then the expression will be evaluated as
(3 + 4) * 5 = 35,
which is incorrect. However, if the multiplication operator () is evaluated first, then the expression will be evaluated as
3 + (4 * 5) = 23,
which is the correct result.
By understanding the precedence order of operators in Python, programmers can write code that is not only correct but also efficient. By using parentheses to group sub-expressions, programmers can control the order of evaluation and ensure that their code is evaluated in the correct order. This can save time and resources and make the code easier to read and maintain.
Furthermore, understanding the precedence order of operators is essential for writing complex expressions in Python. Complex expressions may involve multiple operators and operands, and it is important to know the order in which they will be evaluated to ensure that the expression is evaluated correctly. Failure to understand the precedence order of operators can result in errors that are difficult to debug and fix.
Best Practices for Using Precedence of Operators:
Here are some best practices to keep in mind when using the precedence of operators in Python:
- Always use parentheses to group sub-expressions when the order of evaluation is not clear.
- Use parentheses to improve the readability of complex expressions.
- Avoid using unnecessary parentheses, as they can make the code harder to read.
- Use comments to explain complex expressions.
Conclusion:
The precedence of operators in Python is an important concept to understand for writing correct and efficient code. By following the well-defined order of precedence, you can ensure that your expressions are evaluated correctly. Remember to use parentheses to control the order of evaluation and to improve the readability of your code. With this guide, you should now have a solid understanding of the precedence of operators in Python.
————————————————————————————————————————————————
Exam Time
————————————————————————————————————————————————-
IMPORTANT QUESTIONS : Asked in examinations of Class 12th Computer Science
- What is the order of precedence in Python?
- Which operator is higher precedence in Python?
- What is the correct order of operator precedence?
- Differentiate between operator precedence and associativity in python.
- What is the order of precedence in python from highest to lowest.
- What is the order of precedence in python from highest to lowest.
- Which operator has the highest order of precedence in python.