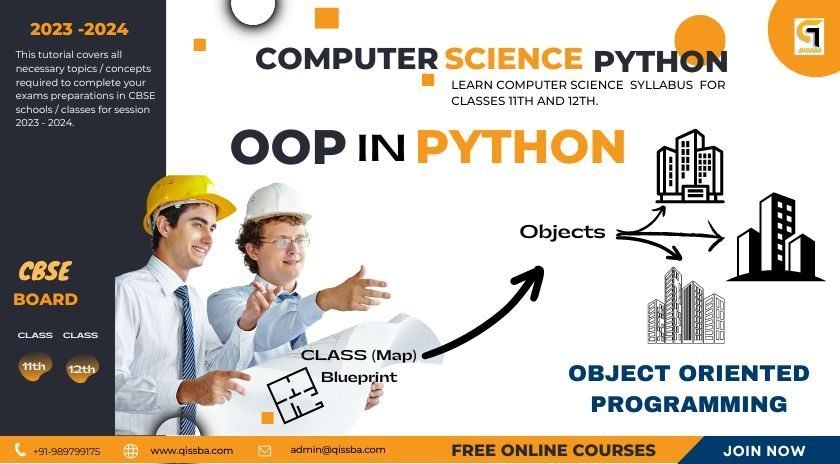
Object Oriented Programming in Python | CBSE – Class 12
In this section of “Object Oriented Programming“, we will provide a comprehensive information about all OOP’s concepts with examples including
- What is Object-Oriented Programming?.
- Concepts of Object-Oriented Programming.
- Is Python Object Oriented Programming Language?.
- How to Implement Object-Oriented Programming in Python?.
- Classes and Objects in Python.
- Abstraction in Python.
- Encapsulation in Python.
- Inheritance in Python.
- Polymorphism in Python.
- Advantages of Abstraction.
- Advantages of Encapsulation.
- Advantages of Inheritance.
- Advantages of Polymorphism.
- Types of Inheritance.
- Types of Polymorphism.
- Overriding V/s Overloading.
and much more, nearly all information about Object-Oriented Programming-OOP which is necessary to become a python Developer / Programming.
And, by the end of this tutorial, readers will have a solid understanding of all about Object-Oriented Programming in Python and will be able to use this knowledge in their own programming projects.
Also this tutorial covers all necessary topics/concepts required to complete your exams preparations in CBSE schools / classes 11th and 12th in 2023 – 2024.
What is Object-Oriented Programming.
In the contemporary software development environment, object-oriented programming (OOP) is a popular programming paradigm that is closely followed by developers. The ideas of OOP enable programmers to create more organised, modular, and reusable computer code, which may assist simplify difficult large-scale applications.
The concept of objects is the foundation of the programming paradigm known as object-oriented programming (OOP). An object is a self-contained entity that is composed of information and behavior. In OOP, classes, which are templates that specify an object’s structure and behavior, are used to build new objects.
Concepts of Object-Oriented Programming
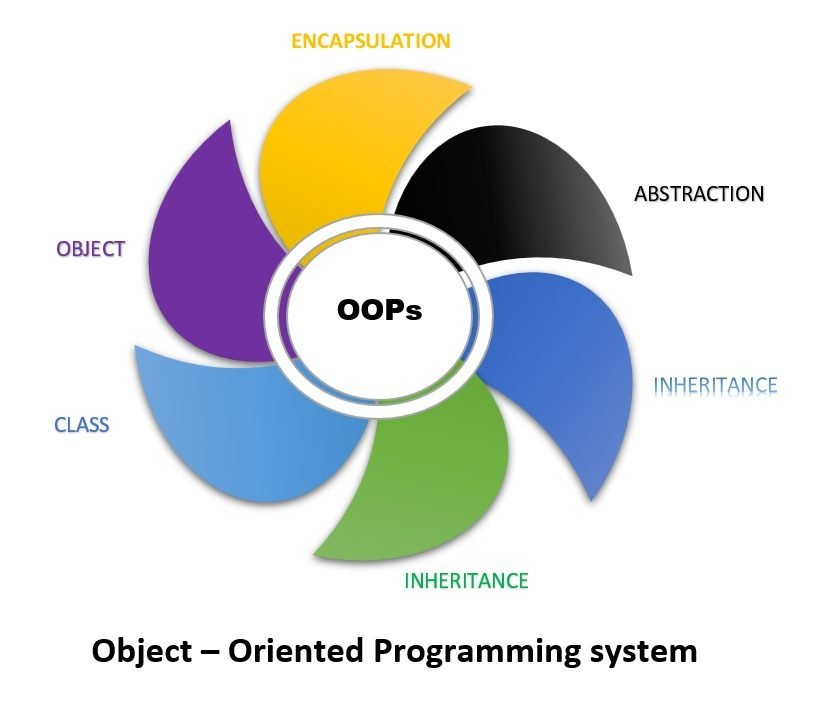
Python is Object Oriented Programming Language.
As we know That the concepts of “object-oriented programming” essentially refers to any programming language that supports all of the concepts described above. And Python supports object-oriented programming by offering features like classes, objects, abstraction, inheritance, polymorphism, and encapsulation, and others. Therefore, we can speak to it as “An Object-Oriented Programming Language”.
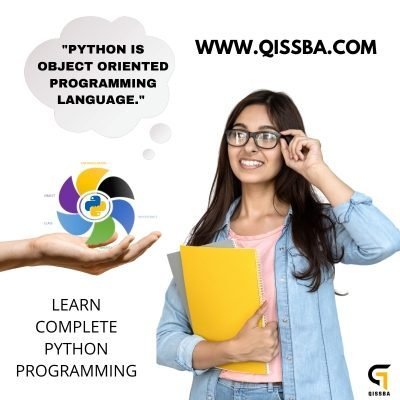
How to Implement Object-Oriented Programming (OOP) in Python
As we mentioned above, to implement Object-Oriented Programming in python, python must be available to create and use the following features of Object-Oriented Programming.
- Classes and Objects
- Abstraction
- Encapsulation
- Inheritance
- Polymorphism
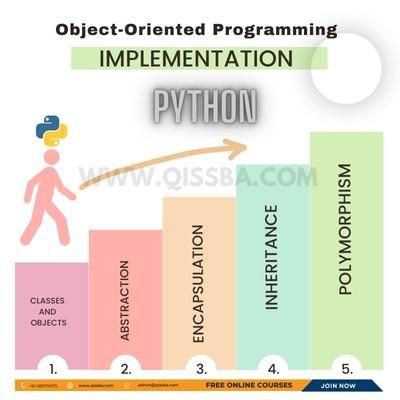
Python is an object-oriented programming language if it supports all the paradigms and allows users to develop Python programmes utilising these capabilities.
Now let’s attempt applying all these OOP concepts to Python, which are a must for any programming language to be considered object-oriented.
Here is an example of object-oriented programming in Python:
class Animal:
def __init__(self, name, species):
self.name = name
self.species = species
def make_sound(self):
print("This animal makes a sound.")
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name, species="Dog")
self.breed = breed
def make_sound(self):
print("Woof!")
class Cat(Animal):
def __init__(self, name, color):
super().__init__(name, species="Cat")
self.color = color
def make_sound(self):
print("Meow!")
dog = Dog("Fido", "Golden Retriever")
cat = Cat("Whiskers", "Tabby")
print(dog.name)
print(dog.species)
print(dog.breed)
dog.make_sound()
print(cat.name)
print(cat.species)
print(cat.color)
cat.make_sound()
The make_sound method outputs a message and establishes an Animal class with a name and a species. Additionally, it defines two classes: Dog and Cat. These classes derive from Animal and feature extra attributes (breed for dogs and colour for cats) as well as methods (make_sound, which prints a unique sound for each kind of animal).
A Dog instance and a Cat instance are created in the main body of the code, and their properties and methods are then accessed and used.
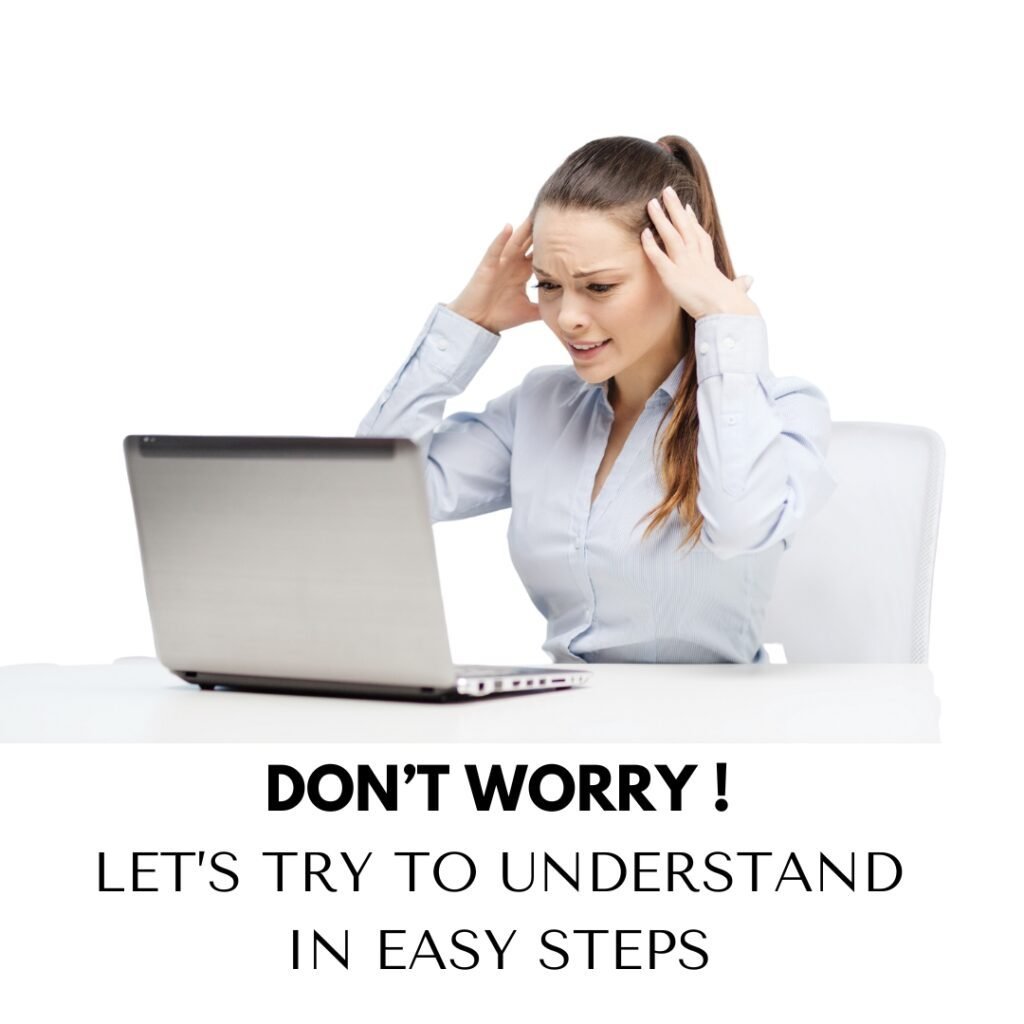
Classes:
In Python, a class is a blueprint for creating objects. It is a user-defined data type that defines a collection of related data and methods. A class is essentially a template that you can use to create multiple instances of objects, each with its own unique state and behaviour.
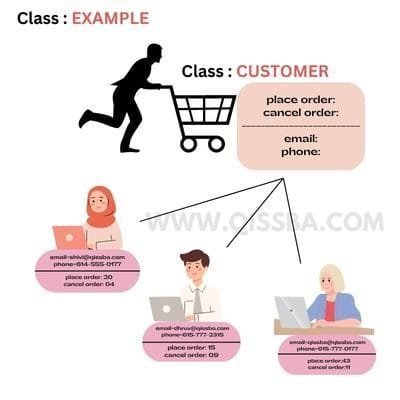
In Python, you can define a class using the “class” keyword, followed by the name of the class and a colon. The properties and methods of the class are defined using indentation, similar to the way that functions are defined in Python.
Here is an example of a simple Python class definition:
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
print(f"Hello, my name is {self.name}.")
This class, called MyClass, has two methods: __init__ and greet. The __init__ method is a special method that is called when an object of the class is created. It initializes the object’s state by setting its name attribute to the value passed as an argument. The greet method is a simple method that prints a message containing the object’s name.
Important: Classes can also have attributes that are shared by all objects of the class. These attributes are defined at the class level and can be accessed using the class name, rather than an instance of the class. For example:
class MyClass:
class_attribute = "This is a class attribute."
def __init__(self, name):
self.name = name
def greet(self):
print(f"Hello, my name is {self.name}.")
In this modified version of the MyClass class, there is a new attribute called class attribute that is defined at the class level. This attribute can be accessed using the class name, as follows:
print(MyClass.class_attribute) # output: "This is a class attribute."
This code prints the value of the class attribute of the MyClass class.
Objects:
In Python, an object is an instance of a class. It is a unique entity that contains data and behaviour defined by the class it was created from. When you create an object from a class, the object is said to be an instance of that class.
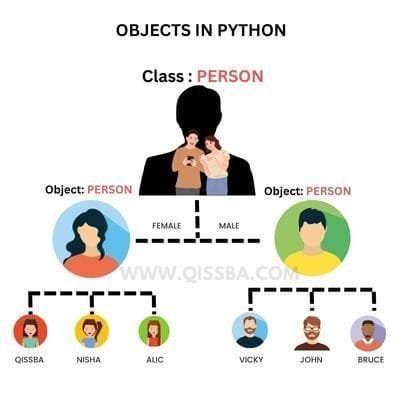
Here is an example of creating an object in Python:
class MyClass:
def __init__(self, name):
self.name = name
obj = MyClass("Alice")
To create an object of this class, you simply call the class like a function, passing any required arguments. For example:
obj = MyClass("Alice")
obj.greet() # output: "Hello, my name is Alice."
This code creates an object of the MyClass class called obj with a name attribute of “Alice”. It then calls the greet method on this object, which prints a greeting message.
Once you have created an object, you can access its attributes and methods using the dot notation. For example, to access the name attribute of the obj object, you can use the following code:
print(obj.name) # output: "Alice"
This code prints the value of the name attribute of the obj object, which is “Alice”.
Important: Objects can also have methods, which are functions that are defined within the class and can be called on instances of the class. For example, if we modify the MyClass class to include a greet method, we can call this method on the obj object as follows:
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
print(f"Hello, my name is {self.name}.")
obj = MyClass("Alice")
obj.greet() # output: "Hello, my name is Alice."
This code defines a greet method that prints a greeting message containing the object’s name. It then creates an object of the MyClass class called obj, and calls the greet method on this object, which prints the message “Hello, my name is Alice.”.
Abstraction:
Abstraction is the process of hiding implementation details while showing only the necessary and relevant information to the user. It is a fundamental concept in Object-Oriented Programming (OOP) that allows us to create simplified models of complex systems. It
In OOP, abstraction is achieved through the use of abstract classes and interfaces. An abstract class is a class that cannot be instantiated and contains at least one abstract method. An abstract method is a method that has no implementation and must be overridden by the subclass. The purpose of an abstract class is to provide a template or blueprint for subclasses to implement.
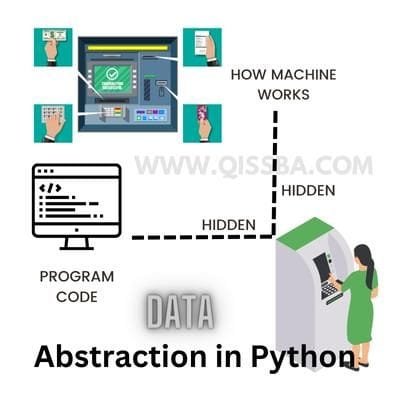
An interface, on the other hand, is a collection of abstract methods that define a contract for a class to implement. Interfaces provide a way to achieve multiple inheritance in Java and other languages that do not support multiple inheritance.
By using abstraction, we can create a layer of separation between the user and the implementation details of a system. This not only makes the code more modular and maintainable, but it also makes it easier to understand and use.
here’s an example of abstraction in Python using abstract classes:
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def speak(self):
pass
class Dog(Animal):
def speak(self):
print("Woof!")
class Cat(Animal):
def speak(self):
print("Meow!")
# The following line will raise an error, as Animal is an abstract class and cannot be instantiated.
a = Animal()
d = Dog()
d.speak() # Output: Woof!
c = Cat()
c.speak() # Output: Meow!
In this example, we define an abstract class Animal that has one abstract method speak(). The Dog and Cat classes are subclasses of Animal and implement the speak() method.
Note that we cannot instantiate the Animal class directly, as it is an abstract class. Instead, we can create instances of the Dog and Cat classes and call their speak() method. This demonstrates how abstraction can be used to define a common interface for different classes, while hiding the implementation details of each individual class.
Advantages of Abstraction:
Abstraction offers several advantages in object-oriented programming. Here are some of the main advantages of abstraction:
- Simplifies code complexity: Abstraction allows you to hide unnecessary details and focus on the essential features of an object or system. This makes it easier to understand, modify, and maintain code.
- Promotes code reusability: By defining a common interface, you can reuse code across different classes and objects. This can save development time and effort.
- Increases flexibility: Abstraction allows you to change the implementation of an object or system without affecting its external interface. This makes it easier to adapt to changing requirements and maintain backward compatibility.
- Reduces dependencies: Abstraction can help you reduce the dependencies between different parts of a system. This can improve the overall reliability and stability of the system.
- Improves code organization: Abstraction encourages you to separate concerns and modularize code. This makes it easier to understand, test, and debug code.
- Facilitates communication: Abstraction provides a common language and vocabulary for discussing complex systems. This can help facilitate communication between developers, designers, and stakeholders.
Encapsulation:
In Python, you can use encapsulation to hide data and behavior within objects, so that they can only be accessed through the object’s public interface. This is achieved using private and protected access modifiers.
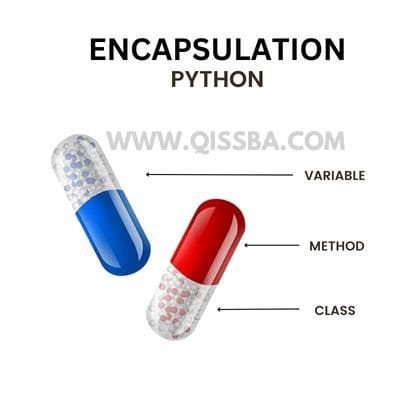
In Python, you can use a single underscore (_) before the name of a property or method to indicate that it should be treated as protected. Protected properties and methods can be accessed from within the class and its subclasses, but not from outside the class.
You can use two underscores (__) before the name of a property or method to indicate that it should be treated as private. Private properties and methods can only be accessed from within the class.
Encapsulation in Python refers to the practice of wrapping data and methods within a single unit, typically a class, and restricting access to the internal components from outside the unit. Encapsulation is one of the fundamental concepts of object-oriented programming (OOP) and is essential for creating robust, secure, and maintainable code.
In Python, encapsulation is achieved by defining the internal components of a class with appropriate access modifiers. By default, all properties and methods of a class are public, meaning they can be accessed from outside the class. However, we can use access modifiers to restrict access to these components as needed. For example:
class MyClass:
def __init__(self):
self.public_property = "This is a public property."
self._protected_property = "This is a protected property."
self.__private_property = "This is a private property."
def public_method(self):
print("This is a public method.")
def _protected_method(self):
print("This is a protected method.")
def __private_method(self):
print("This is a private method.")
Here, public_property and public_method are public, _protected_property and _protected_method are protected, and __private_property and __private_method are private.
Let’s have another example, consider a Person class with name and age properties:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
In this example, both name and age properties are public, meaning they can be accessed from outside the Person class. However, we may want to restrict access to the age property to prevent it from being modified by external code. We can achieve this by using the double underscore prefix to define the property as private:
class Person:
def __init__(self, name, age):
self.name = name
self.__age = age
In this updated example, the age property is now private, and can only be accessed from within the Person class. Any attempt to access or modify the property from outside the class will result in an error:
person = Person("Alice", 30)
print(person.__age)
# This will raise an AttributeError
person.__age = 35
# This will not modify the __age property
By encapsulating the age property within the Person class and making it private, we have improved the security and maintainability of our code. Other components of the Person class, such as methods that manipulate the age property, can still access and modify it as needed, but external code is prevented from doing so.
Advantages of encapsulation:
encapsulation is a fundamental concept in object-oriented programming that plays a crucial role in creating robust, maintainable, and reusable code.
Encapsulation provides several advantages in Python and other object-oriented programming languages:
- Security: By encapsulating internal components, such as properties and methods, we can prevent external code from accessing or modifying them directly. This improves the security and reliability of our code.
- Abstraction: Encapsulation enables us to abstract away the details of how a class is implemented and focus on its public interface. This makes it easier to reason about and use the class in our code.
- Modularity: Encapsulation promotes modularity by allowing us to separate the interface of a class from its implementation. This makes it easier to make changes to the internal implementation of a class without affecting external code that uses the class.
- Code reuse: Encapsulation enables code reuse by allowing us to create objects that have well-defined interfaces and can be used in a variety of contexts.
- Polymorphism: Encapsulation enables polymorphism by allowing us to define a common interface for a set of related classes. This makes it easier to write code that can work with different types of objects in a consistent way.
Inheritance:
Inheritance is a fundamental concept in Object-Oriented Programming (OOP) that allows a new class to be based on an existing class (or multiple existing classes), inheriting its attributes and methods. The existing class is called the base or parent class, and the new class is called the derived or child class.
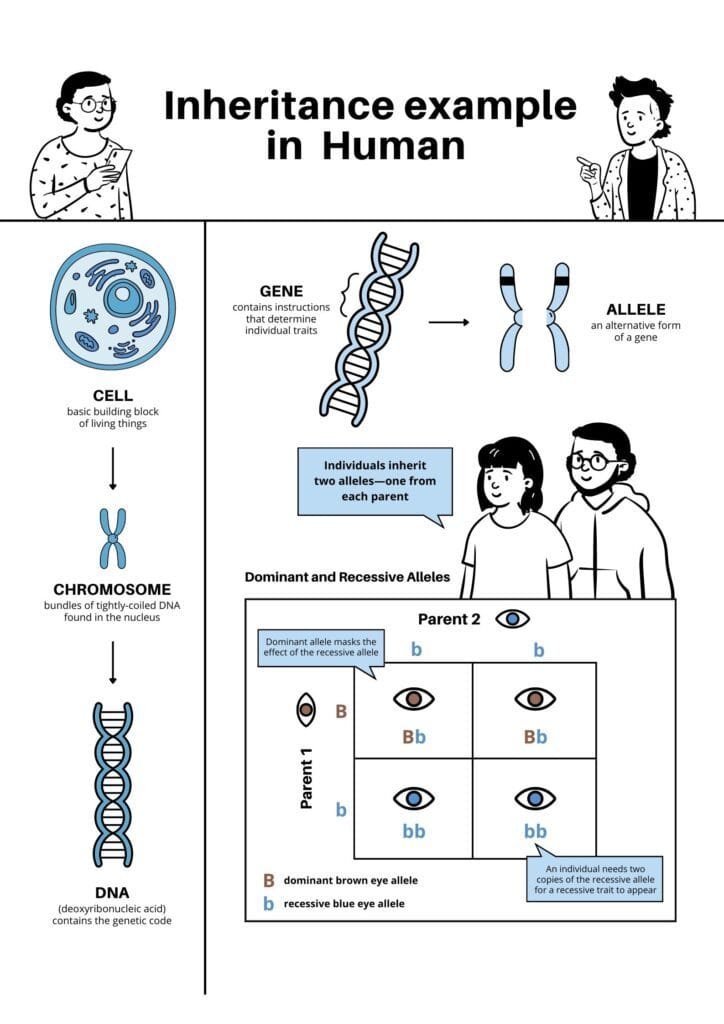
The child class inherits all the non-private attributes and methods of the parent class and can also add its own attributes and methods. This allows for code reuse, as you can create a new class that is similar to an existing class, but with some additional functionality or behavior.
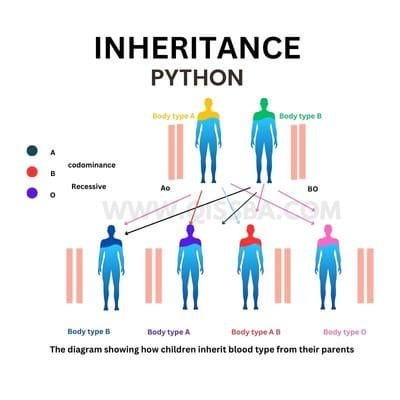
Inheritance is typically used to model a hierarchical relationship between classes. For example, you might have a parent class called Vehicle with attributes and methods common to all vehicles, and then create child classes such as Car, Truck, and Motorcycle that inherit from the Vehicle class and add their own specific attributes and methods.
Inheritance is implemented using the extends or (:) keyword in many object-oriented programming languages such as Java, Python, and C++. The child class can override inherited methods from the parent class to provide its own implementation, and it can also call the parent class’s methods using the super() keyword.
In Python, you can create a new class that inherits properties and methods from an existing class. This is called inheritance. To inherit from a parent class, you simply specify the name of the parent class in the definition of the child class. For example:
class ParentClass:
def parent_method(self):
print("This is a parent method.")
class ChildClass(ParentClass):
def child_method(self):
print("This is a child method.")
Here, ChildClass is a subclass of ParentClass, and it inherits the parent_method method from the parent class. The child_method method is defined in the child class.
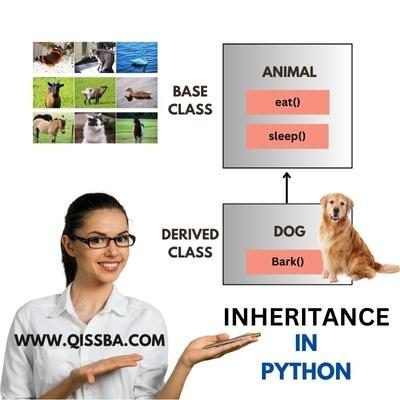
In Python, inheritance is a fundamental feature of Object-Oriented Programming (OOP) that allows a new class to be based on an existing class (or multiple existing classes), inheriting its attributes and methods. Here’s how inheritance is used in Python:
- Defining a Parent Class: First, you define a parent class that contains the common attributes and methods that you want to reuse in the child classes. You can define a parent class using the class keyword.
- Creating a Child Class: Next, you create a child class that inherits from the parent class. To inherit from a parent class, you add the parent class name in parentheses after the child class name.
- Adding New Attributes and Methods: After you have created the child class, you can add new attributes and methods specific to the child class. These attributes and methods will not be available in the parent class.
- Overriding Parent Methods: In some cases, you may want to override a method defined in the parent class in the child class. This can be done by defining a method with the same name in the child class.
Here is an example of how inheritance can be used in Python:
class Animal:
def __init__(self, name, sound):
self.name = name
self.sound = sound
def speak(self):
print(f"{self.name} says {self.sound}")
class Dog(Animal):
def __init__(self, name):
super().__init__(name, "woof")
class Cat(Animal):
def __init__(self, name):
super().__init__(name, "meow")
dog = Dog("Rufus")
dog.speak() # Output: Rufus says woof
cat = Cat("Whiskers")
cat.speak() # Output: Whiskers says meow
In this example, we define a parent class Animal with an __init__() method and a speak() method. We then create two child classes Dog and Cat that inherit from the Animal class and override the sound attribute.
When we create instances of the Dog and Cat classes, they inherit the name and speak() methods from the Animal class, but they override the sound attribute to be “woof” for Dog and “meow” for Cat. When we call the speak() method on the instances, they print out the name and sound of the animal using the overridden sound attribute.
Types of inheritance:
Inheritance is a key feature of Object-Oriented Programming (OOP) that allows one class to inherit properties (attributes and methods) from another class. In Python, there are several types of inheritance that you can use to create a new class from an existing class. The main types of inheritance are:
Single Inheritance:
In single inheritance, a subclass inherits properties from only one parent class.
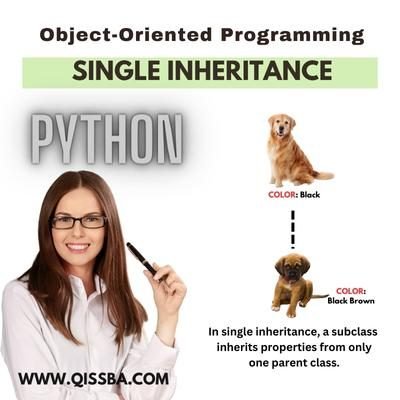
Here is an example of single type of inheritance in Python:
# Single Inheritance
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
print(f"{self.name} speaks")
class Dog(Animal):
def __init__(self, name):
super().__init__(name)
dog = Dog("Rufus")
dog.speak() # Output: Rufus speaks
Multiple Inheritance:
In multiple inheritance, a subclass inherits properties from two or more parent classes.
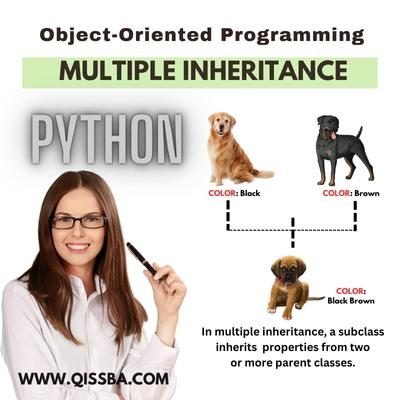
Here is an example of multiple type of inheritance in Python:
# Multiple Inheritance
class A:
def foo(self):
print("A")
class B:
def foo(self):
print("B")
class C(A, B):
pass
c = C()
c.foo() # Output: A
Multi-level Inheritance:
In multi-level inheritance, a subclass inherits properties from a parent class, which in turn inherits from another parent class.
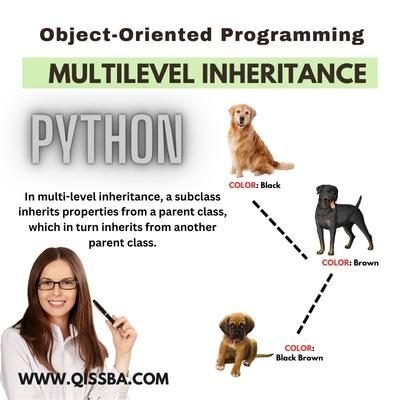
Here is an example of multi-level type of inheritance in Python:
# Multi-level Inheritance
class Animal:
def speak(self):
print("Animal speaks")
class Mammal(Animal):
pass
class Dog(Mammal):
def speak(self):
print("Dog barks")
dog = Dog()
dog.speak() # Output: Dog barks
Hierarchical Inheritance:
In hierarchical inheritance, multiple subclasses inherit properties from a single parent class.
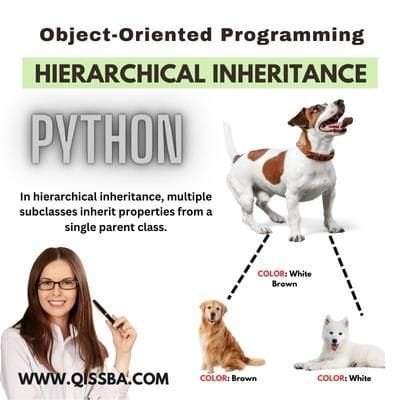
Here is an example of hierarchical type of inheritance in Python:
# Hierarchical Inheritance
class Animal:
def speak(self):
print("Animal speaks")
class Dog(Animal):
def bark(self):
print("Dog barks")
class Cat(Animal):
def meow(self):
print("Cat meows")
dog = Dog()
dog.speak() # Output: Animal speaks
dog.bark() # Output: Dog barks
cat = Cat()
cat.speak() # Output: Animal speaks
cat.meow() # Output: Cat meows
Hybrid Inheritance:
Hybrid inheritance is a combination of multiple inheritance and multi-level inheritance.
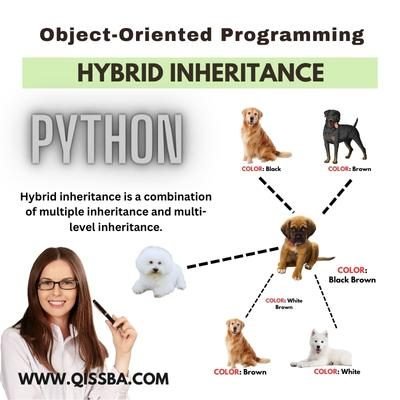
Here is an example of hybrid type of inheritance in Python:
# Hybrid Inheritance
class A:
def foo(self):
print("A")
class B(A):
def foo(self):
print("B")
class C(A):
def foo(self):
print("C")
class D(B, C):
pass
d = D()
d.foo() # Output: B
While discussing above types of Inheritances, we tried to give python code examples for each type of Inheritance.
- In all python code examples, we demonstrate all types of inheritances.
- Single inheritance is shown in the Animal and Dog classes,
- multiple inheritance is shown in the A, B, and C classes,
- multi-level inheritance is shown in the Animal, Mammal, and Dog classes,
- hierarchical inheritance is shown in the Animal, Dog, and Cat classes, and
- hybrid inheritance is shown in the A, B, C, and D classes.
Advantages of Using Inheritance in python:
Inheritance is a powerful object-oriented programming concept in Python that allows one class to inherit properties and behaviour from another class. Here are some advantages of using inheritance in Python:
- Code Reusability: Inheritance allows you to reuse code from existing classes without having to rewrite it. By inheriting from a base class, you can easily add or modify functionality as needed, saving you time and effort.
- Simplified Code: Inheritance can simplify your code by reducing redundancy and promoting modularity. By defining a common set of properties and methods in a base class, you can avoid duplicating code in multiple subclasses.
- Polymorphism: Inheritance enables polymorphism, which allows you to use a single interface to represent multiple types of objects. This can make your code more flexible and adaptable to changing requirements.
- Extensibility: Inheritance makes it easier to extend existing code by adding new functionality to subclasses. This allows you to build on top of existing code without having to modify the base class.
- Improved Maintenance: By using inheritance, you can improve the maintainability of your code by making it easier to understand and modify. By defining common properties and methods in a base class, you can reduce the complexity of individual classes, making it easier to debug and maintain them over time.
Polymorphism:
Polymorphism is a fundamental concept in object-oriented programming that allows objects of different classes to be treated as if they are of the same class. In Python, polymorphism is achieved through the use of inheritance, overloading, and overriding.
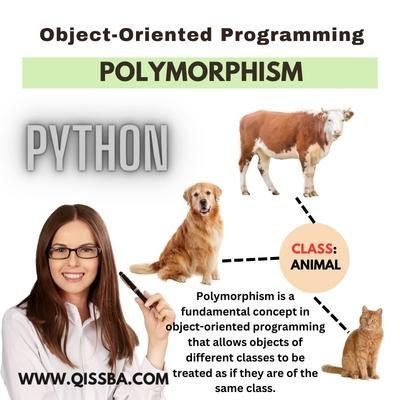
Inheritance allows a subclass to inherit properties and methods from a parent class, and use them as if they were its own. This promotes polymorphism by allowing objects of different classes to be treated as if they are of the same class, as long as they share a common parent class.
Overloading is another way to achieve polymorphism in Python. It allows a single function or method to perform different actions depending on the number or types of arguments passed to it. This means that the same function can be used to perform different tasks depending on the context in which it is used.
Polymorphism is a powerful concept in Python that promotes code reuse, modularity, and flexibility. It allows objects of different classes to be treated as if they are of the same class, and allows for greater customization and flexibility in the design and implementation of software applications.
In Python, you can use polymorphism to write code that can work with objects of different types. Polymorphism is achieved through method overriding and method overloading.
Method overriding is when a child class provides its own implementation of a method that is already defined in the parent class. For example:
class ParentClass:
def print_message(self):
print("This is a message from the parent class.")
class ChildClass(ParentClass):
def print_message(self):
print("This is a message from the child class.")
Here, ChildClass overrides the print_message method of ParentClass.
Here’s another code example of polymorphism in Python using inheritance: Implementation
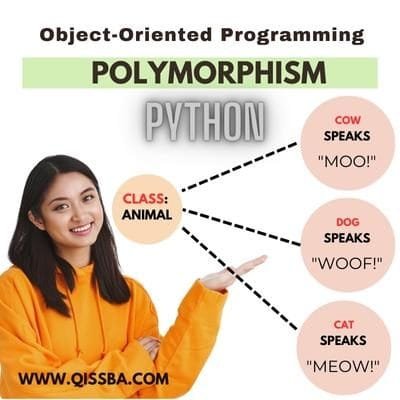
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
class Cow(Animal):
def speak(self):
return "Moo!"
animals = [Dog("Fido"), Cat("Fluffy"), Cow("Bessie")]
for animal in animals:
print(animal.name + " says " + animal.speak())
In this example, we define a base class Animal with a method speak() that does nothing. We then define three subclasses, Dog, Cat, and Cow, each of which overrides the speak() method with its own implementation.
We then create a list of Animal objects, each instantiated as one of the three subclasses. Finally, we iterate over the list and call the speak() method for each object, demonstrating polymorphism in action. Since each subclass overrides the speak() method with its own implementation, we get different output for each animal object, even though they are all of the same base class.
Overriding V/s Overloading:
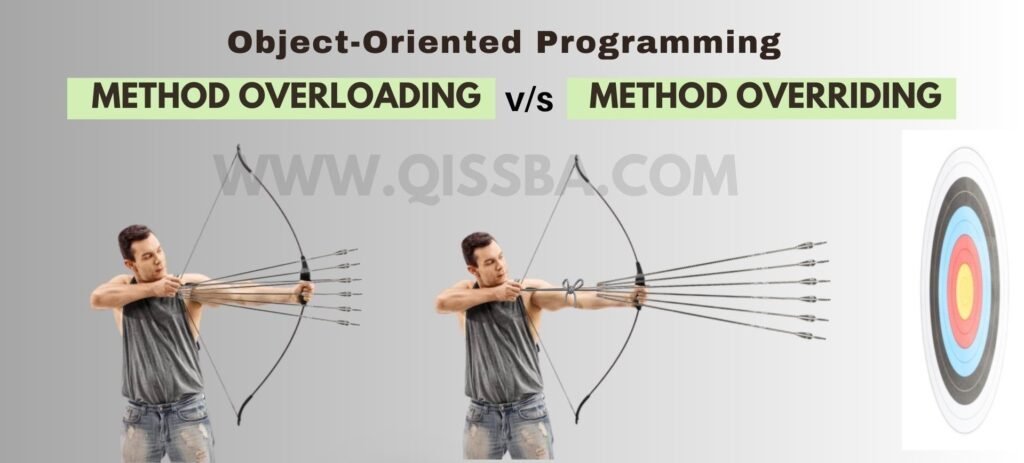
Overriding is the process of defining a method in a subclass that has the same name and arguments as a method in its parent class. By doing this, the subclass can replace or modify the behavior of the inherited method, allowing for greater flexibility and customization.
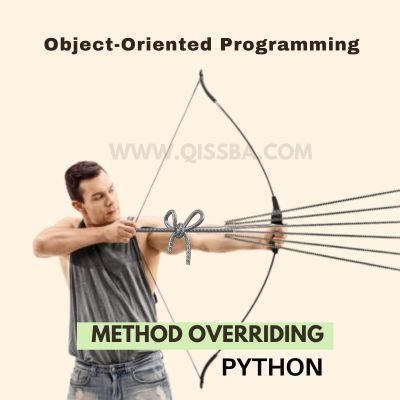
Method overloading is when a class provides multiple methods with the same name, but with different parameters. Python does not support method overloading in the traditional sense, but you can achieve similar functionality using default arguments or variable-length arguments.
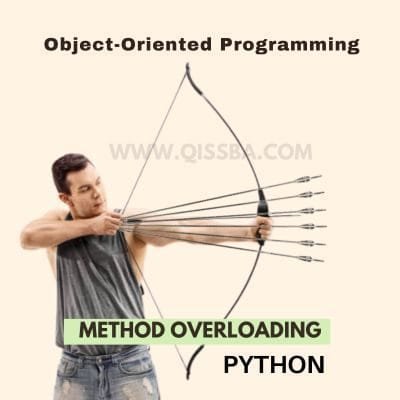
Types of Polymorphism:
Polymorphism is a key concept in object-oriented programming that allows objects of different classes to be treated as if they are of the same class. There are two main types of polymorphism in Python: compile-time polymorphism (also known as static polymorphism) and run-time polymorphism (also known as dynamic polymorphism).
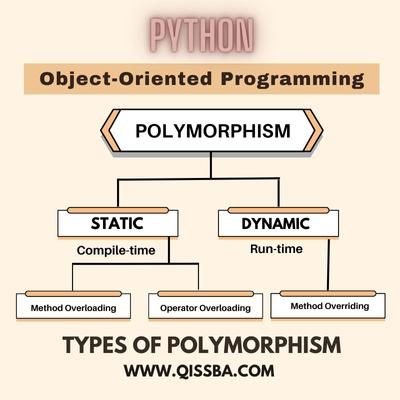
There are two main types of polymorphism in Python:
Compile-time Polymorphism (Static Polymorphism)
Compile-time polymorphism, also known as static polymorphism, is achieved through method overloading. In method overloading, a class can have multiple methods with the same name, but different numbers or types of parameters. The correct method to call is determined at compile-time, based on the types and number of arguments passed to the method. Method overloading is not supported in Python by default, but it can be achieved through the use of default arguments or variable-length argument lists.
Here’s an example of compile-time polymorphism using default arguments:
class MyClass:
def my_method(self, a, b=0, c=0):
return a + b + c
obj = MyClass()
print(obj.my_method(1))
print(obj.my_method(1, 2))
print(obj.my_method(1, 2, 3))
In this example, the my_method() method has three parameters, but the second and third parameters have default values of 0. This allows the method to be called with either one, two, or three arguments, demonstrating compile-time polymorphism.
Run-time Polymorphism (Dynamic Polymorphism)
Run-time polymorphism, also known as dynamic polymorphism, is achieved through method overriding. In method overriding, a subclass can provide its own implementation of a method that is already defined in its parent class. The correct method to call is determined at run-time, based on the actual type of the object. Method overriding is a fundamental feature of object-oriented programming and is supported by default in Python.
Here’s an example of run-time polymorphism using method overriding:
class Animal:
def speak(self):
print("Animal speaks")
class Dog(Animal):
def speak(self):
print("Dog barks")
class Cat(Animal):
def speak(self):
print("Cat meows")
def make_animal_speak(animal):
animal.speak()
dog = Dog()
cat = Cat()
make_animal_speak(dog)
make_animal_speak(cat)
In this example, we define a base class Animal with a speak() method that prints “Animal speaks”. We then define two subclasses, Dog and Cat, each of which overrides the speak() method with its own implementation. Finally, we define a function make_animal_speak() that takes an Animal object as a parameter and calls its speak() method. When we call make_animal_speak() with a Dog object and a Cat object, the correct speak() method is called at runtime, demonstrating run-time polymorphism.
Advantages of Polymorphism:
Polymorphism is a valuable concept in object-oriented programming that enables objects of different classes to be treated as if they were the same type of object. The advantages of polymorphism are numerous, including:
- Code reusability: Polymorphism allows you to reuse code by creating classes that share common methods and properties. This reduces the amount of code you need to write and simplifies maintenance.
- Flexibility: Polymorphism provides flexibility in code design and enables you to create code that is easier to modify and extend. You can create new classes that inherit from existing classes and override or add new methods and properties.
- Encapsulation: Polymorphism can help you to achieve encapsulation, which is the process of hiding the complexity of a program from the user. By using polymorphism, you can create a simplified interface for users to interact with, while hiding the details of the implementation.
- Code organization: Polymorphism can help you to organize your code into smaller, more manageable classes. By creating classes that share common methods and properties, you can keep related code together and make it easier to understand and maintain.
- Code readability: Polymorphism can improve the readability of your code by reducing the amount of repetitive code. By creating classes that share common methods and properties, you can create code that is easier to understand and follow.
How classes and objects are used in OOP?
So far, as we know that the Classes and objects are the fundamental building blocks of object-oriented programming (OOP) in Python. In OOP, you define classes to represent objects, and then create instances of those classes to work with individual objects.
To use classes and objects in Python, you typically follow these steps:
Define a class:
You start by defining a class that specifies the attributes and methods of the object you want to create.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
In this example, we define a Person class with two attributes (name and age) and a method called say_hello that prints a greeting message.
Create objects:
Once you have defined a class, you can create one or more objects (also known as instances) of that class by calling the class constructor.
person1 = Person("Alice", 25)
person2 = Person("Bob", 30)
Here, we create two Person objects called person1 and person2, passing in their name and age as arguments to the constructor.
Use objects:
Once you have created objects, you can use them to access their attributes and call their methods.
print(person1.name) # output: "Alice"
person2.say_hello() # output: "Hello, my name is Bob and I am 30 years old."
Here, we access the name attribute of person1 using the dot notation, and call the say_hello method on person2 to print a greeting message.
Creating a blueprint for an object using classes:
In Python, classes are used to define the blueprint or template for creating objects. Here’s an example that shows how to create a simple class in Python:
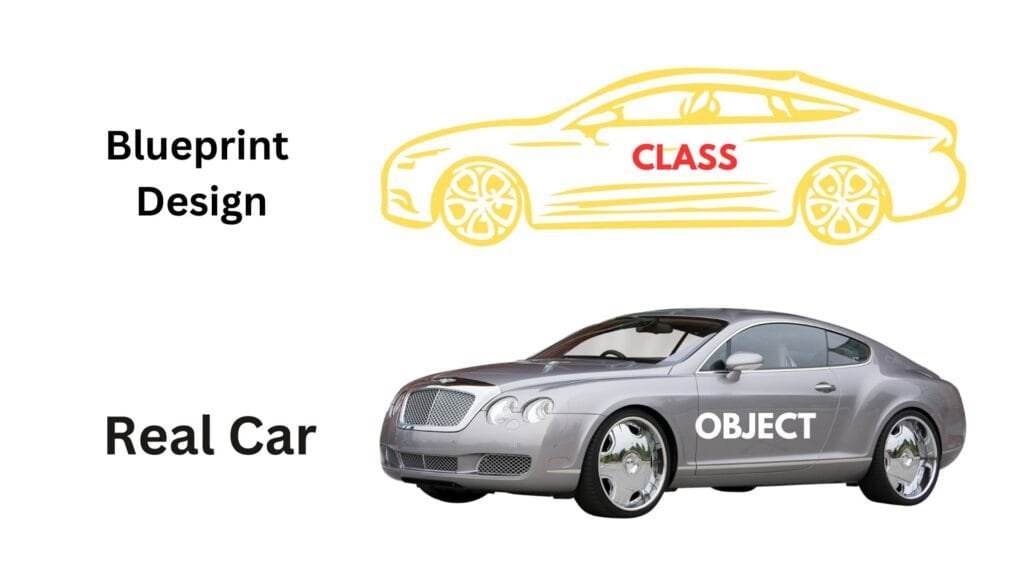
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def drive(self):
print(f"Driving a {self.year} {self.make} {self.model}")
In this example, we define a Car class with three properties: make, model, and year. We also define a method called drive that prints a message indicating that we are driving the car. The __init__ method is a special method in Python classes that is called when an object of the class is created, and is used to initialize the object’s properties with the values passed in as arguments.
To create an object of the Car class, we simply call the class like a function and pass in the values for the properties:
my_car = Car("Toyota", "Corolla", 2020)
This creates a new object called my_car that is an instance of the Car class, with the make, model, and year properties set to “Toyota”, “Corolla”, and 2020, respectively. We can then access the object’s properties and call its methods using dot notation:
print(my_car.make) # output: "Toyota"
my_car.drive() # output: "Driving a 2020 Toyota Corolla"
Differences between classes and objects:
n Python, classes and objects are two distinct concepts that are closely related to each other in the context of object-oriented programming. Here are the key differences between classes and objects in Python:
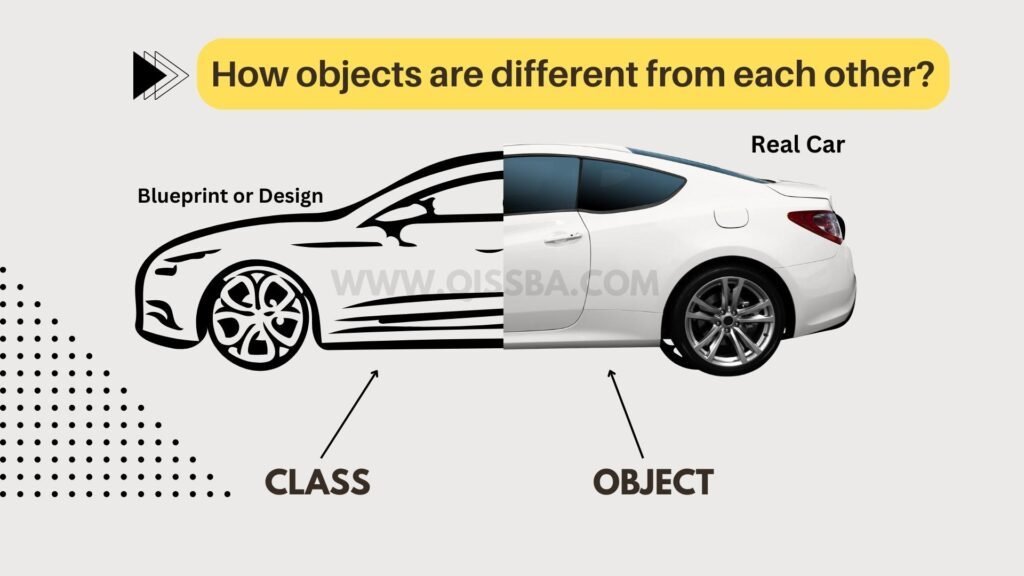
- Definition: A class is a blueprint or a template for creating objects, whereas an object is an instance of a class.
- Properties: A class has properties (also known as attributes), which define the characteristics of the objects created from the class. An object, on the other hand, has values assigned to these properties that define its state.
- Methods: A class can have methods, which are functions that define the behavior of the objects created from the class. An object can call these methods to perform actions or manipulate its state.
- Access: A class can be accessed directly, without the need for an object, whereas an object can only be accessed through its methods or attributes.
- Inheritance: A class can inherit properties and methods from other classes, whereas an object cannot inherit from other objects.
- Memory usage: Classes are stored in memory once and can be used to create multiple objects, which means they are more memory-efficient than creating multiple standalone objects.
- Flexibility: Classes are more flexible than objects, as they can be modified and updated at runtime. You can add or remove properties and methods from a class without affecting existing objects created from it.
- Encapsulation: Classes provide encapsulation, which means that the internal details of a class are hidden from the outside world, and can only be accessed through its methods. This helps to ensure data integrity and security, and prevents accidental modification of internal data.
To support the Above differentiation, here are some examples that illustrates the difference between a class and an object in Python:
Example: 1
# Define a class
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
# Create objects
person1 = Person("Alice", 25)
person2 = Person("Bob", 30)
# Access object attributes
print(person1.name) # output: "Alice"
# Call object methods
person2.say_hello() # output: "Hello, my name is Bob and I am 30 years old."
In this example, we define a Person class with attributes name and age, and a method called say_hello. We then create two Person objects called person1 and person2, and access their attributes and methods. Notice how the class is defined only once, while we create two distinct objects of the same class with different values assigned to their attributes.
Example: 2
Here’s another example that demonstrates how a class and an object differ in their properties and methods:
# Define a class
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
# Create an object
rect = Rectangle(5, 10)
# Access object properties
print(rect.width) # output: 5
# Call object methods
print(rect.area()) # output: 50
In this example, we define a Rectangle class with properties width and height, and a method called area that calculates the area of the rectangle. We then create an object called rect from this class, and access its properties and methods. Notice how the object has values assigned to its properties, while the class only defines the properties and methods that objects of this type will have.
Explanation of the self-keyword:
The self-keyword in Python is used to refer to the instance of a class that is being worked on. It is a special variable that is always the first parameter of a method in a class, and is automatically passed by Python when a method is called on an object. Understanding how to use the self-keyword is essential for working with object-oriented programming in Python, as it allows methods to access the properties and state of an object.
In Python, the self-keyword is used to refer to the instance of a class that is being worked on. It is a special variable that is always the first parameter of a method in a class. When a method is called on an object, Python automatically passes the object itself as the first argument to the method, using the self-keyword.
Here’s an example that demonstrates how the self-keyword works:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
In this example, we define a Person class with two properties: name and age. We also define a method called say_hello that prints a message introducing the person with their name and age.
When we create an object of the Person class, we pass in the values for the name and age properties:
person = Person("John", 30)
We can then call the say_hello method on the object:
person.say_hello()
When the say_hello method is called on the person object, Python automatically passes the person object itself as the first argument to the method, using the self-keyword. This allows the method to access the name and age properties of the object using self.name and self.age, respectively.
OOP’s Properties and Methods in Python:
OOP’s Properties:
Properties are the attributes that belong to an object. They define the state of an object and can be accessed and modified using dot notation. Properties are defined within a class using the def keyword, and can be initialized in the class’s __init__ method. Here’s an example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
In this example, name and age are properties of the Person class. They are initialized in the class’s __init__ method, and can be accessed and modified using dot notation. For example:
person = Person("John", 30)
print(person.name) # output: "John"
person.age = 31
print(person.age) # output: 31
Methods:
Methods are the actions that an object can perform. They define the behavior of an object and can be called using dot notation. Methods are also defined within a class using the def keyword. Here’s an example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
def have_birthday(self):
self.age += 1
In this example, say_hello and have_birthday are methods of the Person class. say_hello prints a message introducing the person with their name and age, while have_birthday increments the person’s age by 1. For example:
person = Person("John", 30)
person.say_hello() # output: "Hello, my name is John and I am 30 years old."
person.have_birthday()
person.say_hello() # output: "Hello, my name is John and I am 31 years old.
Use of the _init_ method:
The __init__ method is a special method in Python that is used to initialize the properties of an object when it is created. The __init__ method is automatically called when an object is instantiated, and can be used to set the initial values of an object’s properties.
Here’s an example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
In this example, the __init__ method takes two parameters, name and age. When a Person object is created, the __init__ method is automatically called with the specified values for name and age. These values are then assigned to the object’s name and age properties using self.name and self.age.
Here’s an example of creating a Person object:
person = Person("John", 30)
person.say_hello()
# output: "Hello, my name is John and I am 30 years old."
In this example, a new Person object is created with the name “John” and age 30. When the say_hello method is called on the person object, it prints a message introducing the person with their name and age.
The __init__ method is a powerful tool in Python that allows you to set the initial state of an object when it is created. It can be used to set default values for properties, validate input, and perform other initialization tasks that are necessary for your program to work correctly.
Creating Objects in Python:
To create objects in Python, follow these steps:
- Define a class: First, you need to define a class that will serve as a blueprint for creating objects. The class defines the properties and methods that objects created from it will have.
- Instantiate the class: Once you have defined a class, you can create instances of that class by instantiating it. To do this, you simply call the class as if it were a function, passing any necessary arguments.
- Access the properties and methods of the object: Once you have created an object, you can access its properties (instance variables) and methods using the dot notation.
Let’s have an explanation of the process of creating objects in Python, following the three steps you mentioned:
Define a class:
A class is a blueprint for creating objects with similar attributes and behaviours. To define a class in Python, use the class keyword, followed by the name of the class and a colon. Inside the class definition, you can define properties (also known as instance variables) and methods (functions that are associated with the class and its instances). Here’s an example:
class Dog:
def __init__(self, name, breed, age):
self.name = name
self.breed = breed
self.age = age
def bark(self):
print("Woof!")
def info(self):
print(f"I am a {self.breed} named {self.name} and I am {self.age} years old.")
In this example, we define a Dog class with three instance variables (name, breed, and age) and two methods (bark and info).
Instantiate the class:
Once you have defined a class, you can create instances of that class by instantiating it. To do this, call the class as if it were a function and pass any necessary arguments. Here’s an example:
my_dog = Dog("Buddy", "Golden Retriever", 2)
This code creates a new instance of the Dog class with the name “Buddy”, the breed “Golden Retriever”, and the age 2. The instance is stored in the variable my_dog.
Access the properties and methods of the object:
Once you have created an object, you can access its properties and methods using the dot notation. Here are some examples:
print(my_dog.name)
# Output: "Buddy"
print(my_dog.age)
# Output: 2
my_dog.bark()
# Output: "Woof!"
my_dog.info()
# Output: "I am a Golden Retriever named Buddy and I am 2 years old."
In this example, we access the name and age instance variables of my_dog, call the bark and info methods of my_dog, and print their output to the console.
That’s the basic process of creating objects in Python! Guys.
Assigning values to object properties:
Assigning values to object properties in Python is done using the self keyword in the class definition. Here’s an example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
In this example, we define a Person class with two properties (name and age) and one method (greet).
To create an instance of this class, we can call it as if it were a function and pass values for the name and age parameters:
person1 = Person("Alice", 30)
This code creates an instance of the Person class with a name of “Alice” and an age of 30, and assigns it to the variable person1.
We can access the properties of the person1 object using dot notation:
print(person1.name) # Output: "Alice"
print(person1.age) # Output: 30
We can also assign new values to the properties of the person1 object using dot notation and the = operator:
person1.name = "Bob"
person1.age = 25
This code assigns a new value of “Bob” to the name property and a new value of 25 to the age property of the person1 object.
We can call the greet method of the person1 object to print a message using its name and age properties:
person1.greet()
# Output: "Hello, my name is Bob and I am 25 years old."
In this example, we call the greet method of person1 to print the message “Hello, my name is Bob and I am 25 years old.” using its name and age properties.
How objects are different from each other?
Objects are different from each other in that they have their own unique set of properties and values, even if they are instances of the same class. This allows for greater flexibility and customization in object-oriented programming.
In object-oriented programming, each object created from a class is unique and has its own set of properties and values. While objects of the same class share the same methods and properties, they can have different values for those properties.
For example, let’s consider a Person class with name and age properties. We can create two instances of this class with different values for name and age:
person1 = Person("Alice", 30)
person2 = Person("Bob", 25)
In this example, person1 and person2 are two separate instances of the Person class, and each has its own unique set of properties and values.
We can access the properties of each object using dot notation:
print(person1.name) # Output: "Alice"
print(person2.name) # Output: "Bob"
In this example, person1 and person2 have different values for the name property.
Similarly, we can call the methods of each object using dot notation:
person1.greet() # Output: "Hello, my name is Alice and I am 30 years old."
person2.greet() # Output: "Hello, my name is Bob and I am 25 years old."
In this example, person1 and person2 have different values for the name and age properties, which are used by the greet method to print a message specific to each object.
Importance of OOP in Modern Python Programming:
Object-oriented programming (OOP) is an important programming paradigm in modern Python programming because it offers several benefits that make code more modular, reusable, and easier to maintain. Here are some of the key reasons why OOP is so important in modern Python programming:
- Encapsulation: OOP allows you to encapsulate data and methods within objects, which can make your code more secure and easier to maintain. By hiding the implementation details of an object and exposing only its interface, you can ensure that the object’s behavior is predictable and consistent.
- Inheritance: OOP supports inheritance, which allows you to create new classes based on existing ones. This can make your code more modular and easier to maintain by promoting code reuse. By inheriting properties and methods from a base class, you can avoid duplicating code and improve the overall design of your program.
- Polymorphism: OOP also supports polymorphism, which allows you to write code that can work with objects of different types in a consistent and predictable way. This can make your code more flexible and adaptable, and can simplify the design of your program.
- Modularity: OOP encourages modularity by allowing you to break down a large program into smaller, more manageable components. By creating classes that encapsulate specific functionality, you can create reusable modules that can be used in multiple programs. This can save you time and effort in the long run, as you can reuse code that you’ve already written rather than starting from scratch.
- Readability: OOP can make your code more readable and understandable by promoting good programming practices such as abstraction, encapsulation, and modularity. By using clear and concise class definitions, you can make your code more self-documenting and easier to understand for other developers who may need to work with your code in the future.
Object-Oriented Programming (OOP) vs Procedural Programming
Object-Oriented Programming (OOP) and Procedural programming are two different programming paradigms with distinct approaches to problem-solving. In the context of Python, the following are some differences between these two types of paradigms:
- Data and behaviour: In procedural programming, data and behaviour are separated, and functions act on the data. In contrast, OOP encapsulates data and behaviour within objects.
- Code organization: Procedural programming is typically organized as a sequence of steps that are executed in order, while OOP is organized around objects that interact with each other.
- Reusability: Procedural programming uses reusable functions, whereas OOP uses reusable objects.
- Abstraction: OOP provides greater abstraction through the creation of abstract classes and interfaces that can be implemented by different objects. Procedural programming does not provide a similar concept of abstraction.
- Inheritance: OOP enables inheritance, allowing classes to inherit properties and behaviors from other classes. Procedural programming does not support inheritance.
- Polymorphism: OOP allows for polymorphism, allowing objects of different classes to be used interchangeably. Procedural programming does not support polymorphism.
- Complexity: OOP is well-suited to handling complex systems with many interrelated parts. In contrast, procedural programming is better suited to smaller programs that do not require complex interactions between functions.
Ultimately, the choice of programming paradigm depends on the nature of the problem being solved and the requirements of the system being built. Python supports both procedural programming and OOP, providing developers with the flexibility to choose the most appropriate paradigm for their needs.
Object-Oriented Programming (OOP) vs Functional Programming:
Object-oriented programming (OOP) and functional programming are two different programming paradigms with distinct approaches to writing code. Here are some key differences between OOP and functional programming:
- Approach: OOP is based on the concept of objects, which encapsulate data and behaviour. Functional programming, on the other hand, is based on functions, which take inputs and produce outputs without modifying state.
- Data: In OOP, data and behaviour are encapsulated within objects. In functional programming, data is typically immutable and passed between functions.
- State: In OOP, objects can have state that can be modified by methods. In functional programming, state is avoided, and functions are pure, meaning that they have no side effects.
- Control flow: In OOP, control flow is typically achieved through method calls and object interactions. In functional programming, control flow is achieved through function calls and function composition.
- Composition: In OOP, composition is achieved through inheritance and composition of objects. In functional programming, composition is achieved through higher-order functions and function composition.
- Concurrency: In OOP, concurrency can be achieved through threads and locks. In functional programming, concurrency can be achieved through immutable data and functional purity.
- Error handling: In OOP, errors are often handled through exceptions and try-catch blocks. In functional programming, errors are handled through returning a value or a result type that indicates an error condition.
In general, OOP is well-suited for building complex systems with many interrelated parts, while functional programming is well-suited for building systems with a focus on data transformation and processing. However, in practice, many modern programming languages, including Python, support both paradigms and allow developers to choose the best approach for their specific problem.
Best Practices and Tips for Python OOP:
Object-oriented programming (OOP) is a popular programming paradigm used in Python, which allows you to organize your code into objects that interact with one another. Here are some best practices and tips for OOP in Python:
- Use inheritance: Inheritance allows you to create new classes that inherit attributes and methods from existing classes. This can help you to reuse code and make your code more modular and easier to maintain.
- Keep classes small: It’s best to keep your classes small and focused on a single task. This makes it easier to understand and maintain your code.
- Use private and protected attributes: Python doesn’t have true private and protected attributes, but you can use naming conventions to indicate which attributes should not be accessed outside of the class. Prefixing an attribute with a single underscore (_) makes it protected, while prefixing with two underscores (__) makes it private.
- Avoid global variables: Global variables can make your code harder to understand and maintain. Instead, use class variables or instance variables to store data that needs to be shared between objects.
- Use properties instead of getters and setters: Properties allow you to define getter and setter methods for your attributes, which can make your code more readable and easier to use.
- Use dunder methods: Dunder (double underscore) methods are special methods in Python that allow you to define behavior for your objects, such as str() for string representation, eq() for equality, and len() for length.
- Use abstract base classes: Abstract base classes (ABCs) provide a way to define interfaces for your classes. This can make it easier to write code that works with multiple types of objects, and helps ensure that your code is well-organized and easy to maintain.
- Write unit tests: Unit tests are an essential part of OOP development. They allow you to verify that your code is working as expected, and make it easier to catch bugs and fix them before they become a problem.
By following these best practices and tips, you can write clean, maintainable, and scalable object-oriented code in Python.
Naming conventions in python with OOP:
Naming conventions are an important aspect of object-oriented programming (OOP) in Python, as they help make your code more readable and maintainable. Here are some commonly used naming conventions in Python for OOP:
- Class names: Class names should be written in CamelCase, with the first letter of each word capitalized. For example, class MyClassName.
- Method names: Method names should be written in lowercase, with words separated by underscores. For example, def my_method_name(self).
- Attribute names: Attribute names should be written in lowercase, with words separated by underscores. For example, self.my_attribute_name.
- Constant names: Constant names should be written in uppercase, with words separated by underscores. For example, MY_CONSTANT_NAME.
- Private attribute and method names: Private attributes and methods should be written with a single underscore prefix. For example, self._my_private_attribute.
- Protected attribute and method names: Protected attributes and methods should be written with a double underscore prefix. For example, self.__my_protected_attribute.
- Module names: Module names should be written in lowercase, with words separated by underscores. For example, my_module_name.py.
By following these naming conventions, you can make your code more consistent and easier to understand for other developers who may work with your code.
Code organization in python with OOP:
Code organization is an important aspect of object-oriented programming (OOP) in Python, as it helps make your code more modular, reusable, and maintainable. Here are some tips for organizing your code with OOP in Python:
- Separate classes into modules: To keep your code organized, it’s a good idea to separate your classes into different modules. Each module should contain related classes that perform a similar task or are part of the same system.
- Use inheritance to reduce code duplication: Inheritance allows you to create a hierarchy of classes that share common attributes and methods. By using inheritance, you can reduce code duplication and make your code more maintainable.
- Use composition to combine classes: Composition allows you to combine multiple classes to create more complex objects. For example, you could create a class for a car that is composed of other classes for the engine, wheels, and transmission.
- Use packages to organize modules: Packages are a way to group related modules together. By using packages, you can organize your code into logical groups, which makes it easier to find and maintain.
- Use abstract base classes to define interfaces: Abstract base classes (ABCs) are a way to define interfaces for your classes. By using ABCs, you can ensure that your classes adhere to a certain set of rules and make it easier to write code that works with multiple types of objects.
- Use docstrings to document your code: Docstrings are a way to document your code and provide information about the purpose of a class, method, or attribute. By using docstrings, you can make your code more understandable and easier to use for other developers.
By following these tips, you can organize your code in a way that is modular, reusable, and easy to maintain, which is essential for any large-scale software project.
Code reuse in python with OOP:
Code reuse is an important aspect of object-oriented programming (OOP) in Python, as it allows you to write code once and reuse it in multiple places, which can save time and reduce code duplication. Here are some tips for code reuse with OOP in Python:
- Inheritance: Inheritance allows you to create a hierarchy of classes that share common attributes and methods. By using inheritance, you can reuse code from a parent class in a child class, which can save time and reduce code duplication.
- Composition: Composition allows you to combine multiple classes to create more complex objects. By using composition, you can reuse code from existing classes by creating new classes that use those classes as components.
- Mixins: Mixins are a way to add functionality to a class without using inheritance. They are typically small classes that define a set of methods that can be added to other classes. By using mixins, you can reuse code across multiple classes without creating a hierarchy of inheritance.
- Function libraries: Function libraries are a collection of functions that can be reused across multiple projects. By using function libraries, you can write code once and use it in multiple projects, which can save time and reduce code duplication.
- External libraries: Python has a large ecosystem of external libraries that can be used to extend the functionality of your code. By using external libraries, you can save time and effort by leveraging code that has already been written and tested by other developers.
By following these tips, you can reuse code in your Python projects and make your code more efficient, maintainable, and scalable.
Choosing the right design pattern in OOP with python:
Choosing the right design pattern is an important aspect of object-oriented programming (OOP) in Python, as it can greatly improve the structure and maintainability of your code. Here are some tips for choosing the right design pattern in OOP with Python:
- Understand the problem: Before choosing a design pattern, you should fully understand the problem you are trying to solve. This will help you identify the requirements and constraints that will guide your choice of design pattern.
- Consider the context: The context of your problem is also important in choosing a design pattern. Consider factors such as the size and complexity of your codebase, the skills and experience of your development team, and the performance requirements of your application.
- Study design patterns: There are many design patterns available, each with its own strengths and weaknesses. Study design patterns to understand their characteristics, trade-offs, and use cases.
- Look for existing solutions: You may be able to find existing solutions to your problem by looking at open-source projects or online resources. This can help you identify patterns that have been used successfully in similar contexts.
- Test and evaluate: Once you have chosen a design pattern, it’s important to test and evaluate its effectiveness. This can help you identify any issues or limitations and make adjustments as needed.
- Some commonly used design patterns in Python include:
- Singleton: A pattern that ensures that only one instance of a class is created and provides global access to that instance.
- Factory: A pattern that provides an interface for creating objects, but allows subclasses to decide which class to instantiate.
- Observer: A pattern that allows an object to notify other objects when its state changes, allowing for loose coupling between objects.
- Decorator: A pattern that allows behaviour to be added to an individual object, either statically or dynamically, without affecting the behaviour of other objects.
- Strategy: A pattern that defines a family of algorithms, encapsulates each one, and makes them interchangeable at runtime.
By considering the problem, context, and available patterns, you can choose the right design pattern to improve the structure and maintainability of your Python code.
Python code for Creating a car class with properties and methods using OOP:
here’s an example Python code for creating a Car class with properties and methods using OOP:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
self.odometer_reading = 0
def get_descriptive_name(self):
long_name = f"{self.year} {self.make} {self.model}"
return long_name.title()
def read_odometer(self):
print(f"This car has {self.odometer_reading} miles on it.")
def update_odometer(self, mileage):
if mileage >= self.odometer_reading:
self.odometer_reading = mileage
else:
print("You can't roll back an odometer!")
def increment_odometer(self, miles):
self.odometer_reading += miles
In this example, the Car class has four properties: make, model, year, and odometer_reading. The __init__ method is used to initialize these properties, with odometer_reading set to 0 by default.
The class also has four methods:
- get_descriptive_name() returns a formatted string that describes the make, model, and year of the car.
- read_odometer() prints the current odometer reading of the car.
- update_odometer(mileage) updates the odometer reading to the given mileage value, as long as it’s greater than or equal to the current reading.
- increment_odometer(miles) increments the odometer reading by the given miles value.
You can create an instance of the Car class and call its methods like this:
my_car = Car('toyota', 'camry', 2021)
print(my_car.get_descriptive_name())
# Output: "2021 Toyota Camry"
my_car.update_odometer(5000)
my_car.read_odometer()
# Output: "This car has 5000 miles on it."
my_car.increment_odometer(100)
my_car.read_odometer()
# Output: "This car has 5100 miles on it."