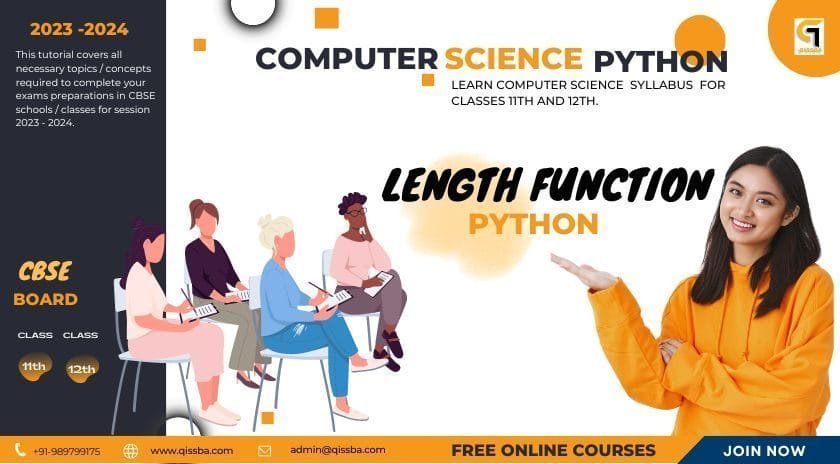
Length Function in Python with Examples | CBSE – Class 12
In this section, “Length Function in Python with Examples ”, we will provide a comprehensive introduction to Length Function in Python – How to use and calculate the length of different objects in python including the following concepts.
- Introduction.
- Syntax and Parameters.
- Examples of Using len() Function.
- Common Errors and Pitfalls.
- Important Questions and Answers.
And, by the end of this tutorial, readers will have a solid understanding of Basics string in Python and will be able to use this knowledge in their own programming projects.
Also this tutorial covers all necessary topics/concepts required to complete your exams preparations in CBSE schools / classes.
Introduction:
Python is a high-level programming language that is widely used in various industries for its simplicity, readability, and vast library support. One of the most commonly used built-in functions in Python is the len() function, which returns the length or size of a given string, list, tuple, dictionary, or any other iterable. In this blog post, we will explain the Python len() function in detail, its syntax and parameters, and provide examples of how to use it in your code. We will also highlight some common errors and pitfalls to avoid while using the function.
What is python len() function?
In Python, len()
is a built-in function that returns the length of an object. The object can be a sequence (like a string, tuple, or list) or a collection (like a dictionary or set). The len()
function calculates the number of elements in the object and returns an integer value. It is a very useful function in Python programming and is used extensively in various applications.
Importance of len() in Python programming
The len() function is a powerful tool in Python programming, which can be used to perform various operations on data structures like strings, lists, tuples, and dictionaries. The len() function is a built-in function in Python, which means that it is available to use without the need for importing any external libraries. The len() function is useful in many different scenarios, including counting the number of characters in a string, finding the number of elements in a list or tuple, and getting the size of a dictionary.
Syntax and Parameters:
The syntax for the len() function is simple and easy to remember. The function takes a single argument, which can be any iterable, and returns the number of elements in that iterable. Here is the syntax for the len() function:
Detailed explanation of the syntax of len() function
The syntax of the len()
function in Python is straightforward and simple. The function takes a single argument, which can be a string, list, tuple, or any other sequence.
Here is the general syntax of the len()
function:
len(sequence)
The sequence
parameter is a mandatory argument that represents the sequence object for which we want to determine the length. The len()
function returns the number of elements in the sequence.
For example, if we want to find the length of a string "Hello, World!"
, we can pass it as an argument to the len()
function as follows:
string = "Hello, World!"
length = len(string)
print("The length of the string is:", length)
The output of the above code will be:
The length of the string is: 13
Similarly, we can use the len()
function to find the length of a list, tuple, or any other sequence object in Python.
Parameters of the function and their usage
The len()
function in Python takes a single parameter, which can be a sequence (such as a string, list, tuple, or dictionary) or a collection (such as a set or frozenset). The parameter is mandatory and must be passed as an argument to the len()
function.
Here are some examples of using different types of parameters with the len()
function:
- String: The
len()
function can be used to determine the length of a string. For example,len("Hello, world!")
would return 13 (the number of characters in the string). - List: The
len()
function can be used to determine the number of elements in a list. For example,len([1, 2, 3, 4, 5])
would return 5 (the number of elements in the list). - Tuple: The
len()
function can also be used to determine the number of elements in a tuple. For example,len((1, 2, 3, 4, 5))
would return 5 (the number of elements in the tuple). - Dictionary: The
len()
function can be used to determine the number of key-value pairs in a dictionary. For example,len({"a": 1, "b": 2, "c": 3})
would return 3 (the number of key-value pairs in the dictionary). - Set: The
len()
function can be used to determine the number of elements in a set. For example,len({1, 2, 3, 4, 5})
would return 5 (the number of elements in the set). - Frozenset: The
len()
function can also be used to determine the number of elements in a frozenset. For example,len(frozenset({1, 2, 3, 4, 5}))
would return 5 (the number of elements in the frozenset).
It is important to note that the len()
function only returns the number of elements in a sequence or collection, and not any additional information about those elements.
Examples of Using len() Function:
Let’s look at some examples of how to use the len() function in Python.
Examples of using len() on a string
In this example, we will use the len() function to find the length of a string.
my_string = "Hello World"
string_length = len(my_string)
print("The length of the string is: ", string_length)
Output: The length of the string is: 11
Examples of using len() on a list
In this example, we will use the len() function to find the length of a list.
my_list = [1, 2, 3, 4, 5]
list_length = len(my_list)
print("The length of the list is: ", list_length)
Output: The length of the list is: 5
Examples of using len() on a tuple
In this example, we will use the len() function to find the length of a tuple.
my_tuple = (1, 2, 3, 4, 5)
tuple_length = len(my_tuple)
print("The length of the tuple is: ", tuple_length)
Output: The length of the tuple is: 5
Examples of using len() on a dictionary
In this example, we will use the len() function to find the number of keys in a dictionary.
my_dict = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
dict_length = len(my_dict)
print("The number of keys in the dictionary is: ", dict_length)
Output: The number of keys in the dictionary is: 3
Common Errors and Pitfalls:
While using the len() function in Python, there are some common errors and pitfalls that you may encounter. Here are a few things to keep in mind:
Common errors encountered while using len() function
TypeError:
If you pass an argument to the len() function that is not a sequence type (e.g., an integer, float, or boolean), you will get a TypeError. For example:
my_int = 123
print(len(my_int))
Output:
TypeError: object of type 'int' has no len()
In this example, we create an integer called my_int
and try to find its length using the len() function. However, since an integer is not a sequence type, we get a TypeError.
ZeroDivisionError:
If you try to divide by the length of a sequence, you may encounter a ZeroDivisionError. For example:
my_list = []
print(10 / len(my_list))
Output:
ZeroDivisionError: division by zero
In this example, we create an empty list called my_list
and try to divide 10 by its length using the len() function. However, since the length of an empty list is 0, we get a ZeroDivisionError.
Pitfalls to avoid while using len() function
While the len()
function is a very useful tool in Python programming, there are some common pitfalls to avoid when using it:
- Passing incorrect arguments: The
len()
function can only be used with objects that have a length. Passing a wrong argument tolen()
can cause unexpected errors. For example, trying to get the length of an integer or a float will result in a TypeError. - Confusing length with index: The
len()
function returns the number of items in an object, not the index of the last item. The index of the last item is always one less than the length of the object. - Inconsistency in the length of nested objects: When dealing with nested objects (like lists inside lists or dictionaries inside dictionaries), the length returned by
len()
may not always be what you expect. This is because the length is calculated based on the number of top-level items in the object, not the total number of items in all levels. - Ignoring the mutability of objects: The
len()
function calculates the length of an object at a specific moment in time. If the object is mutable (like a list), its length can change at any time, so the length returned bylen()
may not be accurate if the object has been modified since the last timelen()
was called.
By being aware of these pitfalls and using the len()
function judiciously, you can avoid common errors and make the most of this powerful Python tool.
Conclusion:
In conclusion, the Python len() function is a powerful and versatile tool that programmers can use to find the size of a string or container object such as a list, tuple, or dictionary. Its simple syntax and ease of use make it a popular choice among Python programmers of all levels.
Throughout this article, we have provided a detailed explanation of the Python len() function, including its syntax and parameters. We have also given numerous examples of how it can be used to find the length of strings, lists, tuples, and dictionaries. Additionally, we have highlighted some common errors and pitfalls to avoid while using the len() function.
It is clear that the len() function is a critical component of the Python programming language, and its applications are wide-ranging. By understanding how to use this function effectively, you can improve your productivity as a Python programmer and streamline your code.
Summary of the key takeaways
In summary, the key takeaways from this article are:
- The len() function is used to find the length of a string or container object in Python.
- The syntax of the len() function is straightforward, with the object to be measured as the input parameter.
- The len() function can be used with many different container objects in Python, including strings, lists, tuples, and dictionaries.
- Understanding common errors and pitfalls associated with using the len() function can help you write more efficient and effective Python code.
Importance of len() function in Python programming
The len()
function is an essential tool for Python programmers when working with data structures that have a length or size. It is a built-in function that is used to determine the length of any given object or sequence in Python. The function returns an integer that represents the number of items or elements present in the object.
The importance of the len()
function lies in its versatility and simplicity. It can be used on a wide range of objects, including strings, lists, tuples, dictionaries, and sets, to name a few. By determining the length of an object, you can gain valuable information about the data you are working with and use it to perform a wide range of operations.
For example, when working with lists, you can use the len()
function to determine the number of items in the list, which can help you with tasks such as indexing and iterating through the list. Similarly, when working with strings, the len()
function can be used to determine the number of characters in the string, which is useful when performing operations such as slicing and concatenation.
The len()
function is also important in data analysis and scientific computing, where it is used to determine the size of arrays and matrices. In addition, it is commonly used in control structures such as loops, where the length of an object is often used as a condition for the loop to terminate.
Future scope and possibilities for len() function in Python.
The len()
function is a fundamental and essential feature of the Python programming language. It is used extensively in various programming applications, and its importance cannot be understated. As Python continues to grow in popularity, so does the use and demand for the len()
function.
In the future, we can expect more enhancements and optimizations to the len()
function in Python. The Python community is constantly working on improving the functionality and performance of this function to make it more efficient and user-friendly.
Moreover, the len()
function is not limited to just strings, lists, tuples, and dictionaries. As Python continues to expand and evolve, we can expect the len()
function to be extended to other data types and structures, making it an even more powerful tool for developers.
Overall, the future of the len()
function in Python is bright, and it will continue to play a crucial role in the world of programming. With its simplicity and versatility, it is a valuable tool for developers of all skill levels and will continue to be an essential part of the Python programming language for years to come.
Exam Time
Here are some important questions with answers for CBSE Board examinations point of view. Also may be helpful for Job interviews.
Question. What is the len() function in Python?
Ans. The len() function is a built-in function in Python that returns
the length of a string, list, tuple, or any other sequence type in Python.
Question. What is the syntax of the len() function?
Ans. The syntax of the len() function is as follows:
len(sequence)
Question. What are the parameters of the len() function?
Ans. The len() function takes only one parameter, which is a sequence type (string, list, tuple, or any other sequence type).
Question. How does the len() function work on a string?
Ans. The len() function returns the number of characters in a string, including whitespaces and special characters.
Question. How does the len() function work on a list or tuple?
Ans. The len() function returns the number of elements in a list or tuple.
Question. Can we use the len() function on a dictionary?
Ans. No, the len() function cannot be used directly on a dictionary. Instead, we can use the len() function on the keys, values, or items of the dictionary to get their lengths.
Question. What are some common errors that can occur while using the len() function?
Ans. Some common errors that can occur while using the len() function are passing an invalid parameter or trying to get the length of a non-sequence type object.
Question. How can we avoid pitfalls while using the len() function?
Ans. To avoid pitfalls while using the len() function, we should ensure that we pass a valid sequence type object to the function and handle exceptions appropriately.
Question. Can the len() function be used to modify the length of a sequence?
Ans. No, the len() function only returns the length of a sequence and cannot be used to modify the length of a sequence.
Question. How can we use the len() function to check if a sequence is empty?
Ans. We can use the len() function to check if a sequence is empty by comparing the length of the sequence with 0. If the length is 0, then the sequence is empty.
Question. Can we use the len() function on custom sequence types?
Ans. Yes, we can use the len() function on custom sequence types as long as they implement the len() method.
Question. What is the time complexity of the len() function?
Ans. The time complexity of the len() function is O(1) as it simply returns the length of a sequence without iterating over the elements.
Question. How does the len() function work with nested sequences?
Ans. The len() function returns the length of the outermost sequence when used with nested sequences.
Question. Can we use the len() function on a set or a frozenset?
Ans. Yes, we can use the len() function on a set or a frozenset as they are sequence types in Python.
Question. How can we use the len() function to iterate over a sequence?
Ans. We cannot use the len() function to iterate over a sequence directly. Instead, we can use a for loop to iterate over the sequence using the len() function to get the length of the sequence.
Question. How does the len() function handle sequences with non-integer indices?
Ans. The len() function returns the length of a sequence based on the number of elements in the sequence, regardless of the indices used to access them.
Question. How can we use the len() function to find the length of a string?
Ans. We can use the len() function to find the length of a string by passing the string as an argument to the len() function.
Question. How can we use the len() function to find the number of elements in a list of lists?
Ans. We can use the len() function to find the number of elements in a list of lists by iterating over the outer list and calling the len() function on each inner list, and then summing the results.
Question. Can we use the len() function on a dictionary?
Ans. No, we cannot use the len() function on a dictionary directly. Instead, we can use the len() function on the keys(), values(), or items() methods of the dictionary to get the number of keys, values, or key-value pairs, respectively.
Question. Can we use the len() function on a tuple?
Ans. Yes, we can use the len() function on a tuple as it is a sequence type in Python.
Question. What is the time complexity of the len() function in Python?
Ans. The time complexity of the len() function in Python is O(1) for most data types, including strings, lists, tuples, and sets. However, for dictionaries, the time complexity is O(n) where n is the number of key-value pairs in the dictionary.
Question. How can we use the len() function to check if a string is empty?
Ans. We can use the len() function to check if a string is empty by checking if the length of the string is equal to zero. For example, if len(string) == 0, the string is empty.
Question. Can we use the len() function to find the length of a file?
Ans. No, we cannot use the len() function to find the length of a file directly. Instead, we need to use other functions in Python, such as os.path.getsize(), to find the size of the file in bytes.
Question. How can we use the len() function with user-defined objects in Python?
Ans. To use the len() function with user-defined objects in Python, we need to define the len() method in the class definition. This method should return the number of elements in the object, which can be used by the len() function.
Question. How can we use the len() function to find the length of a 2D array in Python?
Ans. We can use the len() function to find the length of a 2D array in Python by using the len() function on the outer list, which returns the number of rows in the array. We can then use the len() function on each inner list to get the number of columns in the array.
Question. Can we use the len() function to find the length of a string with Unicode characters?
Ans. Yes, we can use the len() function to find the length of a string with Unicode characters. The len() function returns the number of characters in the string, including Unicode characters.
Question. Can we use the len() function to find the length of a string with escape characters?
Ans. Yes, we can use the len() function to find the length of a string with escape characters. The len() function returns the number of characters in the string, including escape characters.
Question. How can we use the len() function with a range object in Python?
Ans. We can use the len() function with a range object in Python by converting the range object to a list and then using the len() function on the list. For example, len(list(range(1, 10))) returns 9.
Question. How can we use the len() function to find the number of elements in a tuple?
Ans. We can use the len() function to find the number of elements in a tuple by using the len() function on the tuple. For example, len((1, 2, 3, 4)) returns 4.
Question. How can we use the len() function to find the number of unique elements in a list in Python?
Ans. We can use the len() function with the set() function to find the number of unique elements in a list in Python. For example, len(set([1, 2, 3, 3, 4, 4, 4, 5])) returns 5.