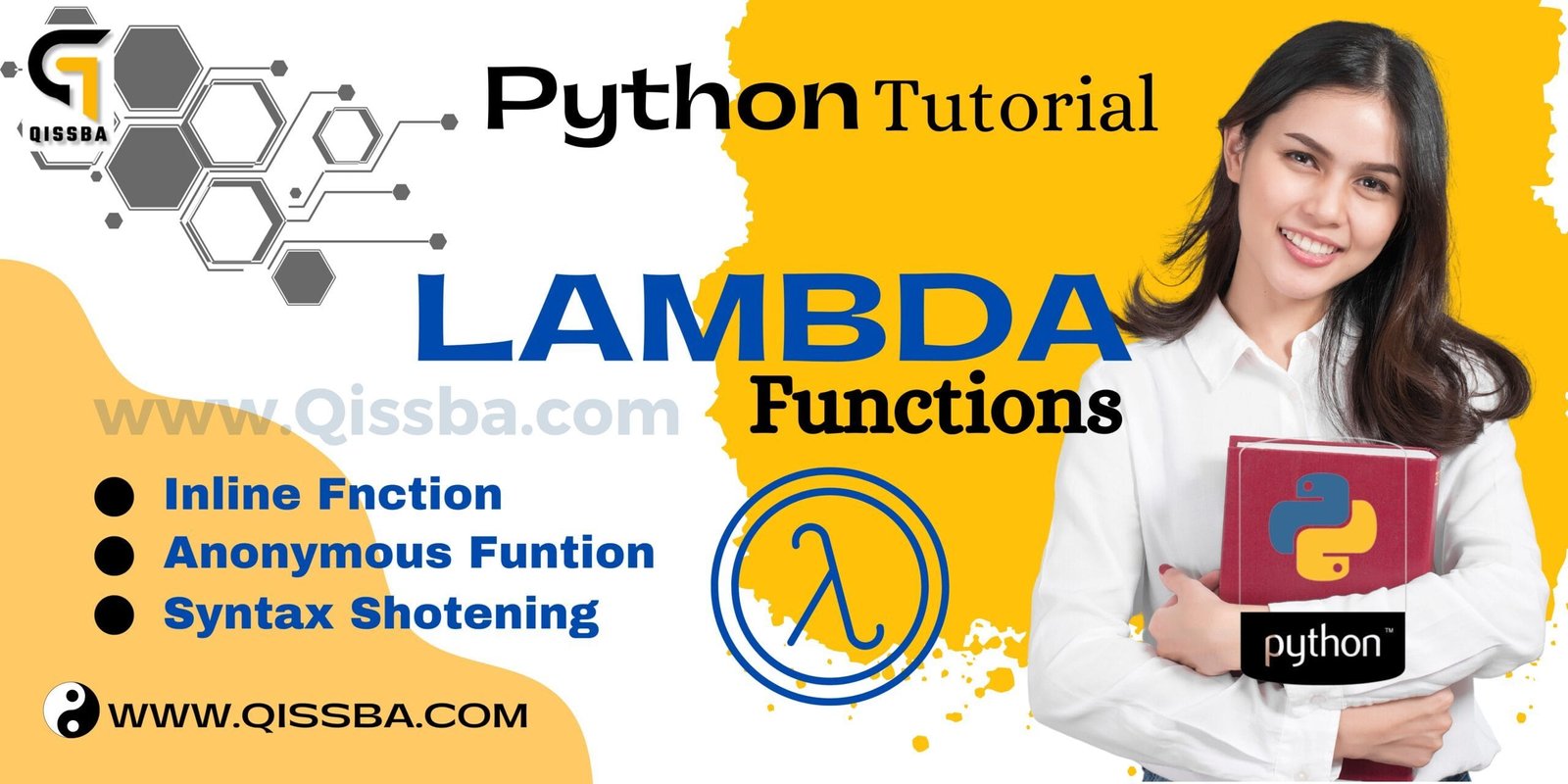
Lambda Function in Python | CBSE – Class 12
Lambda Functions in Python
Introduction:
Lambda functions in Python, also known as anonymous functions, are a powerful feature in Python that allow you to create simple functions without a name. They are often used in functional programming to write shorter and more concise code. In this post, we will explain what lambda functions are, how to use them, and provide examples of their use.
What are Lambda Functions?
A lambda function in Python is a small, anonymous function that can have any number of arguments, but can only have one expression. Lambda functions are defined using the lambda
keyword, followed by the function’s arguments and a colon, then the expression to be evaluated. Here’s an example of a lambda function:
multiply = lambda x, y: x * y
In this example, multiply
is a lambda function that takes two arguments, x
and y
, and returns their product.
How does lambda function work?
In Python, a lambda function is a small, anonymous function that can be used in place of a named function. Lambda functions are created using the lambda keyword, followed by one or more arguments, a colon, and an expression that is evaluated and returned when the lambda function is called.
Here is the basic syntax of a lambda function in Python:
lambda arguments: expression
The arguments are the inputs to the function, and the expression is the output. For example, here is a simple lambda function that takes a single argument and returns its square:
square = lambda x: x**2
This lambda function takes a single argument x
, squares it using the **
operator, and returns the result. This lambda function can be called like a regular function:
result = square(5)
print(result) # Output: 25
Lambda functions can also be used as arguments to other functions. For example, the built-in map()
function takes a function and applies it to each element of a sequence. Here is an example that uses a lambda function to square each element of a list:
numbers = [1, 2, 3, 4, 5]
squares = map(lambda x: x**2, numbers)
print(list(squares)) # Output: [1, 4, 9, 16, 25]
In this example, the lambda function is passed as the first argument to the map()
function. The lambda function takes a single argument x
and squares it using the **
operator. The map()
function applies this lambda function to each element of the numbers
list and returns a new list with the squared values.
How to Use Lambda Functions?
Lambda functions can be used in many situations where you would normally define a function. One common use case is to pass them as arguments to other functions. Here’s an example of using a lambda function as an argument to the sorted()
function:
fruits = ['banana', 'apple', 'orange', 'cherry']
sorted_fruits = sorted(fruits, key=lambda fruit: fruit.lower())
print(sorted_fruits)
In this example, we sort the fruits
list in alphabetical order, ignoring case. The key
argument of the sorted()
function is a lambda function that takes a fruit as input and returns its lowercase version.
Use Cases for Lambda Functions
Lambda functions are commonly used in situations where a small, one-line function is needed. Here are a few examples:
- Sorting a list of tuples based on the second element:
my_list = [(1, 2), (4, 1), (3, 6), (5, 3)]
sorted_list = sorted(my_list, key=lambda x: x[1])
- Filtering a list based on a condition:
my_list = [1, 2, 3, 4, 5, 6]
filtered_list = list(filter(lambda x: x % 2 == 0, my_list))
- Mapping a list of values to a new list:
my_list = [1, 2, 3, 4, 5]
mapped_list = list(map(lambda x: x**2, my_list))
Advantages of Lambda Functions
One advantage of lambda functions is that they allow you to write shorter and more concise code. They are also useful when you need to pass a simple function as an argument to another function, without defining a named function. Another advantage is that they can make your code more readable, by making it clear that you are defining a function to be used in a specific context.
Key Benefits of Lambda
One advantage of using lambda functions in Python is that they can make your code more concise and readable. Because lambda functions are anonymous and can be defined in place, they can eliminate the need for creating separate functions for small tasks.
Another advantage is that lambda functions can be used as arguments to other functions, which can make your code more modular and easier to read.
Conclusion
Lambda functions are a powerful and flexible feature in Python that can help you write more concise and readable code. They are particularly useful in functional programming, but can be used in many other situations as well. With the examples and information provided in this post, you should have a good understanding of how to use lambda functions in your Python code.
————————————————————————————————————————————–
Exam Time
Here are a few commonly asked questions related to Lambda Functions in Python:
1. What is the difference between a lambda function and a regular function in Python?
A lambda function is an anonymous function that is defined on a single line and typically used for short and simple operations. A regular function is a named function that can have multiple lines of code and can be called multiple times throughout the code.
2. How do you pass multiple arguments to a lambda function?
You can pass multiple arguments to a lambda function by separating them with commas. Here’s an example:
sum = lambda x, y, z: x + y + z
result = sum(1, 2, 3)
print(result) # Output: 6
3. Can you use lambda functions in object-oriented programming in Python?
Yes, you can use lambda functions in object-oriented programming in Python. For example, you can define a lambda function as a method of a class and call it like any other method.
4. When should you use a lambda function instead of a regular function in Python?
You should use a lambda function instead of a regular function when you need to define a simple function for a specific purpose and don’t want to define a named function. Lambda functions are particularly useful when you need to pass a function as an argument to another function, as they allow you to define the function inline.
5. What are some common use cases for lambda functions in Python?
Lambda functions are commonly used in functional programming and in situations where you need to define a short, simple function for a specific purpose. Some common use cases for lambda functions include sorting, filtering, mapping, and reducing data.
6. Can you use a lambda function as a key function when sorting data in Python?
Yes, you can use a lambda function as a key function when sorting data in Python. The key function is used to transform each element of the iterable before sorting, and a lambda function can be used to define the transformation.
7. How do you define a lambda function that takes no arguments in Python?
To define a lambda function that takes no arguments in Python, you can use an empty set of parentheses like this:
greeting = lambda:
"Hello, world!"
print(greeting()) # Output: "Hello, world!"
8. How do you use a lambda function to filter data in Python?
To use a lambda function to filter data in Python, you can pass the lambda function as an argument to the built-in filter()
function. Here’s an example:
numbers = [1, 2, 3, 4, 5]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4]
9. What is the scope of variables defined inside a lambda function in Python?
Variables defined inside a lambda function in Python have local scope, which means they are only accessible within the lambda function itself. Variables defined outside the lambda function have global scope and can be accessed within the lambda function if they are passed as arguments.
10. How do you use a lambda function to sort data in Python?
To use a lambda function to sort data in Python, you can pass the lambda function as the key
argument to the built-in sorted()
function or the sort()
method of a list. Here’s an example:
fruits = ["apple", "banana", "cherry", "durian"]
sorted_fruits = sorted(fruits, key=lambda fruit: len(fruit))
print(sorted_fruits) # Output: ["apple", "banana", "cherry", "durian"]
11. Why use lambda function in Python?
Lambda functions in Python are a way to create small, anonymous functions that can be used as arguments to other functions or used in expressions. There are several reasons why you might choose to use lambda functions in your Python code:
- Conciseness: Lambda functions are a way to write small, simple functions in a more concise way than using the def keyword. This can make your code easier to read and understand.
- Readability: Lambda functions can be easier to read in certain situations, especially when used as arguments to other functions. For example, if you are passing a simple function to a sorting function, using a lambda function can make the code more readable.
- Functionality: Lambda functions can be used to create complex expressions that can be used in a variety of ways. For example, you can use a lambda function to create a filter for a list comprehension.
- Efficiency: In some cases, using a lambda function can be more efficient than creating a regular function. This is because lambda functions are evaluated at runtime, so they do not need to be compiled and saved to memory.
- Flexibility: Lambda functions can be used in a variety of contexts, from simple one-liners to more complex expressions. This flexibility can make your code more versatile and adaptable to different situations.
12. Why is it called lambda function in Python?
The term “lambda function” comes from lambda calculus, a mathematical system developed by Alonzo Church in the 1930s. In lambda calculus, a lambda abstraction is a function definition that does not have a name. Instead, it consists of a lambda symbol (λ), followed by a variable, a dot (.), and an expression. The variable represents the parameter of the function, and the expression represents the body of the function.
Lambda functions in Python are similar in that they are anonymous functions that can take arguments and return a value. They are created using the lambda keyword, which is derived from the lambda symbol used in lambda calculus. In fact, the syntax of lambda functions in Python closely mirrors the syntax of lambda abstractions in lambda calculus.
Although the term “lambda function” is not unique to Python, it is commonly used in the Python community to refer to anonymous functions created using the lambda keyword. These functions are often used in functional programming and in situations where a small, one-line function is needed.
————————————————————————————————————————————–
Are you a student of CBSE Board for Classes 11th and 12th,
Do you want to learn Complete Python Programming
Try our free Computer Science with Python Courses for free